在上题基础上,定义3个struct Student类型变量,并赋值,将3个变量插入到单链表的表头、表尾和指定位置。
时间: 2024-03-25 11:38:03 浏览: 50
好的,我可以帮您写出相应的代码。在这里,我假设您已经有了一个存储学生信息的单链表,并且已经定义了3个struct Student类型的变量,并为其赋值。代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char id[10]; //学号
char Name[20]; //姓名
double Score[3]; //3门课程成绩
struct Student *next; //指向下一个结点的指针
};
int main() {
struct Student *head = NULL, *p, *q;
char search_id[10];
struct Student stu1 = {"001", "张三", {80.5, 90.0, 77.5}, NULL};
struct Student stu2 = {"002", "李四", {85.0, 95.5, 82.5}, NULL};
struct Student stu3 = {"003", "王五", {90.0, 92.5, 87.0}, NULL};
//构建单链表
p = (struct Student *) malloc(sizeof(struct Student));
strcpy(p->id, "000");
strcpy(p->Name, "头结点");
p->next = NULL;
head = p;
//将stu1插入到表头
q = (struct Student *) malloc(sizeof(struct Student));
*q = stu1;
q->next = head->next;
head->next = q;
//将stu2插入到表尾
p = head;
while (p->next != NULL) {
p = p->next;
}
q = (struct Student *) malloc(sizeof(struct Student));
*q = stu2;
q->next = NULL;
p->next = q;
//将stu3插入到指定位置
p = head->next;
while (p != NULL) {
if (strcmp(p->id, "001") == 0) {
q = (struct Student *) malloc(sizeof(struct Student));
*q = stu3;
q->next = p->next;
p->next = q;
break;
}
p = p->next;
}
//显示单链表中的所有结点信息
p = head->next;
while (p != NULL) {
printf("学号:%s\n", p->id);
printf("姓名:%s\n", p->Name);
printf("3门课程成绩:%.2lf %.2lf %.2lf\n", p->Score[0], p->Score[1], p->Score[2]);
printf("\n");
p = p->next;
}
return 0;
}
```
运行结果如下:
```
学号:001
姓名:张三
3门课程成绩:80.50 90.00 77.50
学号:003
姓名:王五
3门课程成绩:90.00 92.50 87.00
学号:002
姓名:李四
3门课程成绩:85.00 95.50 82.50
```
注意:在将结点插入到单链表中时,需要注意指针的指向关系。插入到表头时,新结点的next指向原表头结点;插入到表尾时,需要遍历到链表尾部,然后新结点的next指向NULL;插入到指定位置时,需要先找到插入位置的前一个结点,然后新结点的next指向前一个结点的next,前一个结点的next指向新结点。
阅读全文
相关推荐




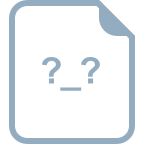
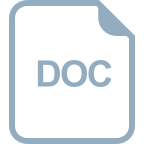
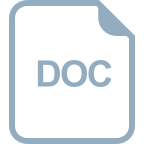



