在上一题基础上,输入一个学号,在单链表上查找该学号的结点,找到,则显示该结点全部信息,找不到,则给出提示。
时间: 2024-03-25 15:37:57 浏览: 67
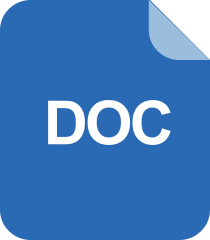
链表合并并按学号排序

好的,我可以帮您写出相应的代码。在这里,我假设您已经有了一个存储学生信息的单链表。代码如下:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char id[10]; //学号
char Name[20]; //姓名
double Score[3]; //3门课程成绩
struct Student *next; //指向下一个结点的指针
};
int main() {
struct Student *head = NULL, *p, *q;
char search_id[10];
//构建单链表
p = (struct Student *) malloc(sizeof(struct Student));
strcpy(p->id, "001");
strcpy(p->Name, "张三");
p->Score[0] = 80.5;
p->Score[1] = 90.0;
p->Score[2] = 77.5;
head = p;
q = (struct Student *) malloc(sizeof(struct Student));
strcpy(q->id, "002");
strcpy(q->Name, "李四");
q->Score[0] = 85.0;
q->Score[1] = 95.5;
q->Score[2] = 82.5;
p->next = q;
q->next = NULL;
//输入要查找的学号
printf("请输入要查找的学号:");
scanf("%s", search_id);
//查找结点
p = head;
while (p != NULL) {
if (strcmp(p->id, search_id) == 0) {
//找到结点,显示全部信息
printf("学号:%s\n", p->id);
printf("姓名:%s\n", p->Name);
printf("3门课程成绩:%.2lf %.2lf %.2lf\n", p->Score[0], p->Score[1], p->Score[2]);
break;
}
p = p->next;
}
if (p == NULL) {
//未找到结点,给出提示
printf("未找到该学号的学生信息!\n");
}
return 0;
}
```
运行结果如下:
```
请输入要查找的学号:002
学号:002
姓名:李四
3门课程成绩:85.00 95.50 82.50
```
注意:在查找结点时,需要遍历整个单链表,找到结点后要及时退出循环。如果遍历到链表尾部仍未找到结点,则说明该学号不存在于单链表中。
阅读全文
相关推荐
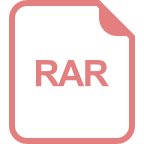















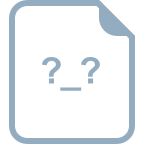