用c语言帮我写一个fir滤波器,告诉我怎么用
时间: 2023-08-16 16:42:47 浏览: 49
好的,下面是一个简单的C语言实现的FIR滤波器代码,以及使用方法:
```c
#include <stdio.h>
#include <stdlib.h>
#define N 21 // FIR滤波器阶数
#define Fs 8000 // 采样率
float h[N] = {0.045, -0.052, -0.035, 0.0089, 0.046, 0.032, -0.027, -0.051, -0.0048, 0.072, 0.1, 0.072, -0.0048, -0.051, -0.027, 0.032, 0.046, 0.0089, -0.035, -0.052, 0.045}; // FIR滤波器系数
int main() {
// 读取输入信号
FILE *fpin = fopen("input.pcm", "rb");
if (fpin == NULL) {
printf("Failed to open input file!\n");
return -1;
}
fseek(fpin, 0, SEEK_END);
int len = ftell(fpin) / sizeof(short);
fseek(fpin, 0, SEEK_SET);
short *input = (short *)malloc(len * sizeof(short));
fread(input, sizeof(short), len, fpin);
fclose(fpin);
// FIR滤波
short *output = (short *)malloc(len * sizeof(short));
for (int i = 0; i < len; i++) {
float temp = 0.0;
for (int j = 0; j < N; j++) {
if (i - j >= 0) {
temp += h[j] * input[i - j];
}
}
output[i] = (short)temp;
}
// 写入输出信号
FILE *fpout = fopen("output.pcm", "wb");
if (fpout == NULL) {
printf("Failed to open output file!\n");
return -1;
}
fwrite(output, sizeof(short), len, fpout);
fclose(fpout);
free(input);
free(output);
return 0;
}
```
使用方法:
1. 将需要滤波的原始信号保存为一个二进制的PCM文件(采样率为Fs,量化位数为16位)。
2. 将上述代码复制到一个C文件中,并将FIR滤波器的系数(即h数组)修改为你需要的系数。
3. 编译运行该C文件,处理后的输出信号将保存为一个二进制的PCM文件(采样率为Fs,量化位数为16位)。
注意事项:
1. FIR滤波器的系数需要根据实际需求进行设计。
2. 由于FIR滤波器是一种线性时不变系统,因此可以先将输入信号分段处理,再将多个处理结果拼接起来,以减少内存占用。
相关推荐
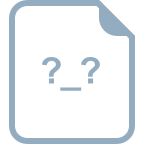
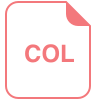
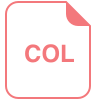
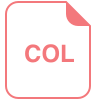
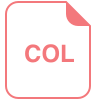
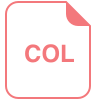









