广义感知器参数学习算法用python实现
时间: 2023-08-10 20:05:02 浏览: 55
好的,广义感知器算法也是一种二分类算法,它可以处理线性不可分的数据。下面是这个算法的伪代码:
```
输入:训练数据集D={(x1,y1),(x2,y2),...,(xn,yn)},其中xi∈R^n,yi∈{+1,-1}
输出:感知器模型f(x)
1. 初始化权重向量w0,偏置b0,学习率η,迭代次数T
2. for t=1,2,...,T do
3. for i=1,2,...,n do
4. if yi(wt*xi+b)≤0 then
5. wt+1=wt+η*yi*phi(xi)
6. bt+1=bt+η*yi
7. end if
8. end for
9. end for
10. f(x)=sign(w*x+b)
其中,phi(xi)是一个非线性函数,可以是多项式函数、高斯函数等。
下面是使用Python实现的代码:
```python
import numpy as np
class GeneralizedPerceptron:
def __init__(self, learning_rate=1, max_iter=10, phi=None):
self.lr = learning_rate
self.max_iter = max_iter
self.phi = phi if phi else (lambda x: x)
def fit(self, X, y):
n_samples, n_features = X.shape
self.w = np.zeros(n_features)
self.b = 0
for t in range(self.max_iter):
for i in range(n_samples):
if y[i] * (np.dot(self.w, self.phi(X[i])) + self.b) <= 0:
self.w += self.lr * y[i] * self.phi(X[i])
self.b += self.lr * y[i]
def predict(self, X):
return np.sign(np.dot(self.phi(X), self.w) + self.b)
```
这里的phi(xi)默认为恒等函数,即phi(xi)=xi。如果需要使用其他的非线性函数,可以在初始化时传入一个函数对象。例如,如果要使用多项式函数,可以这样定义:
```python
def polynomial(x, degree=2):
n_samples, n_features = x.shape
x_poly = x
for d in range(2, degree+1):
x_poly = np.concatenate((x_poly, x**d), axis=1)
return x_poly
gp = GeneralizedPerceptron(phi=polynomial)
```
这里定义了一个名为polynomial的函数,用于将输入的数据集进行多项式变换。在初始化GeneralizedPerceptron对象时,将该函数作为phi参数传入即可。
相关推荐
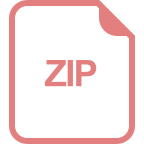
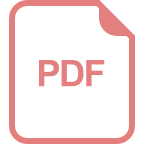
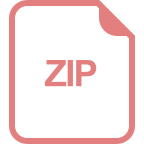














