用C语言写有两个有序链表A和B,A中存放的是1 ,3,5,7,9。 B中存放的是2,4,6,8,10. 要求将两个有序链表合成一个有序链表1,2,3,4,5,6,7,8,9,10
时间: 2023-09-12 15:12:12 浏览: 121
好的,以下是C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* mergeTwoLists(struct ListNode* l1, struct ListNode* l2){
struct ListNode *dummy = (struct ListNode*)malloc(sizeof(struct ListNode)); // 创建虚拟头节点
struct ListNode *cur = dummy; // cur指针指向新链表的最后一个节点,初始指向虚拟头节点
while (l1 && l2) {
if (l1->val < l2->val) {
cur->next = l1;
l1 = l1->next;
} else {
cur->next = l2;
l2 = l2->next;
}
cur = cur->next;
}
cur->next = l1 ? l1 : l2; // 如果l1或l2还有剩余节点,直接将其添加到新链表的末尾
return dummy->next; // 返回新链表的头节点
}
int main() {
struct ListNode a[5] = {{1, NULL}, {3, NULL}, {5, NULL}, {7, NULL}, {9, NULL}}; // 创建链表A
struct ListNode b[5] = {{2, NULL}, {4, NULL}, {6, NULL}, {8, NULL}, {10, NULL}}; // 创建链表B
for (int i = 0; i < 4; i++) {
a[i].next = &a[i+1];
b[i].next = &b[i+1];
}
struct ListNode *head = mergeTwoLists(a, b); // 合并两个有序链表
while (head) { // 遍历新链表并输出
printf("%d ", head->val);
head = head->next;
}
return 0;
}
```
在这个实现中,我们使用了结构体来表示链表节点,其中val表示节点的值,next表示指向下一个节点的指针。在main函数中,我们首先创建了两个有序链表A和B,然后使用for循环将它们连接成链表。最后,调用mergeTwoLists函数合并两个有序链表,并将新链表的头节点赋值给head指针。最后,我们再次遍历新链表并输出其中的节点值。
阅读全文
相关推荐
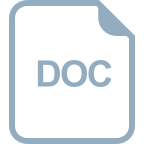
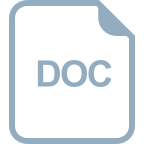
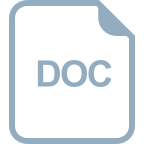















