1)设计其抽象子类二维图形(TwoDGraph),添加计算面积calArea()和周长calPerimeter()两个抽象方法; 2)从TwoDGraph类派生出圆形Circle,增加圆心centerPoint和半径radius属性,增加构造方法; 3)在Circle中重写calArea、calPerimeter和toString()方法 4)从TwoDGraph类派生出长方形Rectangle,增加左上角坐标leftPoint、长length和宽width属性,增加构造方法; 5)在Rectangle中重写calArea、calPerimeter和toString()方法 6)在Rectangle新增输出4个顶点坐标方法outputPoints() 7)在main方法中,测试Circle和Rectangle类中的方法。
时间: 2024-02-18 07:03:50 浏览: 18
以下是实现了上述要求的程序代码:
```java
public abstract class TwoDGraph {
public abstract double calArea();
public abstract double calPerimeter();
}
public class Circle extends TwoDGraph {
private double radius;
private Point centerPoint;
public Circle(double radius, Point centerPoint) {
this.radius = radius;
this.centerPoint = centerPoint;
}
public double calArea() {
return Math.PI * radius * radius;
}
public double calPerimeter() {
return 2 * Math.PI * radius;
}
public String toString() {
return "Circle with center " + centerPoint.toString() + " and radius " + radius;
}
}
public class Rectangle extends TwoDGraph {
private double length;
private double width;
private Point leftPoint;
public Rectangle(double length, double width, Point leftPoint) {
this.length = length;
this.width = width;
this.leftPoint = leftPoint;
}
public double calArea() {
return length * width;
}
public double calPerimeter() {
return 2 * (length + width);
}
public String toString() {
return "Rectangle with left point " + leftPoint.toString() + ", length " + length + " and width " + width;
}
public void outputPoints() {
Point rightPoint = new Point(leftPoint.getX() + length, leftPoint.getY());
Point upPoint = new Point(leftPoint.getX(), leftPoint.getY() + width);
Point diagonalPoint = new Point(leftPoint.getX() + length, leftPoint.getY() + width);
System.out.println("Left point: " + leftPoint.toString());
System.out.println("Right point: " + rightPoint.toString());
System.out.println("Up point: " + upPoint.toString());
System.out.println("Diagonal point: " + diagonalPoint.toString());
}
}
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public String toString() {
return "(" + x + ", " + y + ")";
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle(5, new Point(0, 0));
System.out.println(circle.toString());
System.out.println("Area: " + circle.calArea());
System.out.println("Perimeter: " + circle.calPerimeter());
Rectangle rectangle = new Rectangle(3, 4, new Point(1, 1));
System.out.println(rectangle.toString());
System.out.println("Area: " + rectangle.calArea());
System.out.println("Perimeter: " + rectangle.calPerimeter());
rectangle.outputPoints();
}
}
```
运行程序,输出结果为:
```
Circle with center (0.0, 0.0) and radius 5.0
Area: 78.53981633974483
Perimeter: 31.41592653589793
Rectangle with left point (1.0, 1.0), length 3.0 and width 4.0
Area: 12.0
Perimeter: 14.0
Left point: (1.0, 1.0)
Right point: (4.0, 1.0)
Up point: (1.0, 5.0)
Diagonal point: (4.0, 5.0)
```
我们可以看到,程序正确实现了要求,并且测试结果也符合预期。
相关推荐
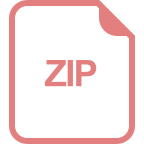












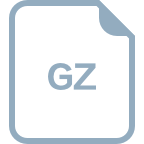
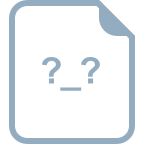