编写一个程序实现复数的四则运算
时间: 2024-09-19 18:05:08 浏览: 36
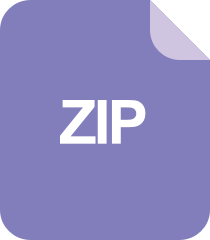
用C语言实现复数的四则运算
在 C++ 中,我们可以定义一个结构体或类来表示复数,包括实部 (real) 和虚部 (imaginary),然后实现加、减、乘、除这四个基本的数学运算。这里我将展示一个简单的复数类实现:
```cpp
#include <iostream>
class Complex {
public:
// 定义复数的构造函数
Complex(double real = 0, double imag = 0)
: real(real), imag(imag) {}
// 获取/设置实部和虚部
double getReal() const { return real; }
void setReal(double r) { real = r; }
double getImaginary() const { return imag; }
void setImaginary(double i) { imag = i; }
// 复数运算方法(加法)
Complex add(Complex other) {
return Complex(real + other.real, imag + other.imag);
}
// 减法
Complex subtract(Complex other) {
return Complex(real - other.real, imag - other.imag);
}
// 乘法
Complex multiply(Complex other) {
return Complex(real * other.real - imag * other.imag,
real * other.imag + imag * other.real);
}
// 除法(假设除数不为零)
Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
return Complex((real * other.real + imag * other.imag) / denominator,
(imag * other.real - real * other.imag) / denominator);
}
private:
double real, imag; // 实部和虚部
};
int main() {
Complex c1(3, 4); // 创建复数 c1 = 3 + 4i
Complex c2(1, 2); // 创建复数 c2 = 1 + 2i
Complex sum = c1.add(c2);
Complex difference = c1.subtract(c2);
Complex product = c1.multiply(c2);
Complex quotient = c1.divide(c2);
std::cout << "Sum: (" << sum.getReal() << ", " << sum.getImaginary() << ")\n";
std::cout << "Difference: (" << difference.getReal() << ", " << difference.getImaginary() << ")\n";
std::cout << "Product: (" << product.getReal() << ", " << product.getImaginary() << ")\n";
std::cout << "Quotient: (" << quotient.getReal() << ", " << quotient.getImaginary() << ")\n";
return 0;
}
```
这个程序首先创建了两个复数 `c1` 和 `c2`,然后分别进行了加、减、乘、除操作,并输出结果。
阅读全文
相关推荐
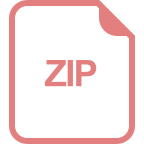
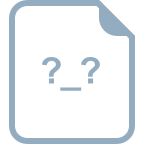
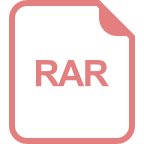
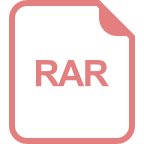
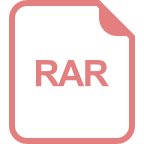
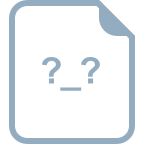
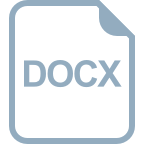
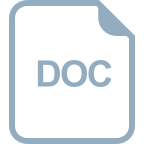
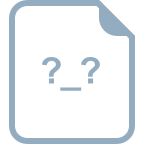
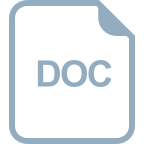
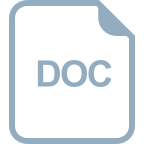
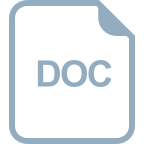
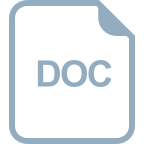


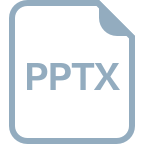