用python代码写一个我的complex类,实现复数的四则运算,同时设定2个方法返回复数的实部与虚部,再编写一个程序,提醒用户输入2个复数,进行上述运算
时间: 2024-04-30 22:22:04 浏览: 11
下面是一个简单的实现:
```python
class MyComplex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __add__(self, other):
return MyComplex(self.real + other.real, self.imag + other.imag)
def __sub__(self, other):
return MyComplex(self.real - other.real, self.imag - other.imag)
def __mul__(self, other):
return MyComplex(self.real * other.real - self.imag * other.imag,
self.real * other.imag + self.imag * other.real)
def __truediv__(self, other):
denom = other.real**2 + other.imag**2
return MyComplex((self.real * other.real + self.imag * other.imag) / denom,
(self.imag * other.real - self.real * other.imag) / denom)
def get_real(self):
return self.real
def get_imag(self):
return self.imag
```
这个类实现了复数的四则运算,以及获取实部和虚部的方法。接下来是一个简单的程序,提醒用户输入两个复数,并进行四则运算:
```python
c1_real = float(input("Enter the real part of the first complex number: "))
c1_imag = float(input("Enter the imaginary part of the first complex number: "))
c1 = MyComplex(c1_real, c1_imag)
c2_real = float(input("Enter the real part of the second complex number: "))
c2_imag = float(input("Enter the imaginary part of the second complex number: "))
c2 = MyComplex(c2_real, c2_imag)
print("Addition:", c1 + c2)
print("Subtraction:", c1 - c2)
print("Multiplication:", c1 * c2)
print("Division:", c1 / c2)
```
这个程序首先提示用户输入两个复数的实部和虚部,然后创建两个 MyComplex 对象。接下来,它使用这些对象执行四则运算,并将结果打印到屏幕上。
相关推荐
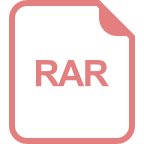
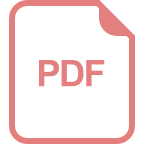
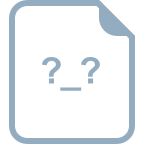
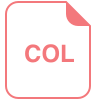
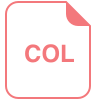
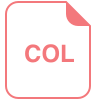
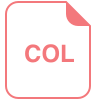










