请你帮我写一下这份代码
时间: 2023-08-28 11:26:43 浏览: 55
好的,下面是一份基于C语言和EasyX图形库的祖玛游戏代码,实现了您所要求的功能,供参考:
```c
#include <graphics.h>
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <time.h>
#define WIDTH 640 // 游戏窗口的宽度
#define HEIGHT 480 // 游戏窗口的高度
#define RADIUS 16 // 祖玛球的半径
#define SPEED 6 // 祖玛球的速度
#define COLORS 4 // 祖玛球的颜色数
#define ROWS 9 // 祖玛球的行数
#define COLS 15 // 祖玛球的列数
// 定义祖玛球的颜色
enum Color {
RED,
YELLOW,
GREEN,
BLUE
};
// 定义祖玛球的结构体
struct Ball {
int x, y; // 祖玛球的坐标
int color; // 祖玛球的颜色
struct Ball* prev; // 祖玛球的前一个节点
struct Ball* next; // 祖玛球的后一个节点
};
// 定义全局变量
int score = 0; // 得分
int sound = 1; // 是否播放背景音乐
struct Ball* head = NULL; // 祖玛球链的头节点
struct Ball* tail = NULL; // 祖玛球链的尾节点
int launch_x = WIDTH / 2; // 发射球的初始位置
int launch_y = HEIGHT - RADIUS; // 发射球的初始位置
int launch_dx = 0; // 发射球的水平速度
int launch_dy = -SPEED; // 发射球的垂直速度
int launch_color = RED; // 发射球的颜色
int gameover = 0; // 游戏是否结束
// 初始化祖玛球链
void init_balls() {
int i, j;
struct Ball* ball = NULL;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
ball = (struct Ball*)malloc(sizeof(struct Ball));
ball->x = (j + 1) * RADIUS * 2;
ball->y = (i + 1) * RADIUS * 2;
ball->color = rand() % COLORS;
ball->prev = NULL;
ball->next = NULL;
if (head == NULL) {
head = ball;
tail = ball;
} else {
tail->next = ball;
ball->prev = tail;
tail = ball;
}
}
}
}
// 绘制祖玛球链
void draw_balls() {
struct Ball* ball = head;
while (ball != NULL) {
setfillcolor(ball->color == RED ? RED : WHITE);
setlinecolor(BLACK);
fillcircle(ball->x, ball->y, RADIUS);
drawcircle(ball->x, ball->y, RADIUS);
ball = ball->next;
}
}
// 绘制发射球
void draw_launch() {
setfillcolor(launch_color == RED ? RED : WHITE);
setlinecolor(BLACK);
fillcircle(launch_x, launch_y, RADIUS);
drawcircle(launch_x, launch_y, RADIUS);
}
// 更新祖玛球链的位置和状态
void update_balls() {
struct Ball* ball = head;
while (ball != NULL) {
ball->x += SPEED;
if (ball->x + RADIUS > WIDTH) {
ball->x = WIDTH - RADIUS;
SPEED = -SPEED;
} else if (ball->x - RADIUS < 0) {
ball->x = RADIUS;
SPEED = -SPEED;
}
ball = ball->next;
}
}
// 更新发射球的位置和状态
void update_launch() {
launch_x += launch_dx;
launch_y += launch_dy;
if (launch_x + RADIUS > WIDTH) {
launch_x = WIDTH - RADIUS;
launch_dx = -launch_dx;
} else if (launch_x - RADIUS < 0) {
launch_x = RADIUS;
launch_dx = -launch_dx;
}
if (launch_y - RADIUS < 0) {
launch_y = RADIUS;
launch_dy = -launch_dy;
}
}
// 检测发射球是否碰到祖玛球链
void check_collision() {
struct Ball* ball = head;
while (ball != NULL) {
if ((launch_x - ball->x) * (launch_x - ball->x) +
(launch_y - ball->y) * (launch_y - ball->y) <= RADIUS * RADIUS) {
launch_dx = 0;
launch_dy = 0;
launch_color = ball->color;
ball->color = launch_color;
struct Ball* temp = ball->next;
while (temp != NULL && temp->color == launch_color) {
temp = temp->next;
}
if (temp != NULL) {
temp->prev->next = tail->next;
tail->next = ball;
ball->prev->next = temp;
temp->prev = ball->prev;
ball->prev = tail;
tail = temp->prev;
while (tail->prev != NULL && tail->prev->color == launch_color) {
tail = tail->prev;
}
} else {
tail->next = ball;
ball->prev = tail;
tail = ball;
}
launch_x = WIDTH / 2;
launch_y = HEIGHT - RADIUS;
launch_dx = 0;
launch_dy = -SPEED;
break;
}
ball = ball->next;
}
}
// 检测是否有连续的同色球
int check_chain(struct Ball* ball) {
int count = 1;
struct Ball* prev = ball->prev;
struct Ball* next = ball->next;
while (prev != NULL && prev->color == ball->color) {
count++;
prev = prev->prev;
}
while (next != NULL && next->color == ball->color) {
count++;
next = next->next;
}
return count >= 3;
}
// 递归消除同色球
void remove_balls(struct Ball* ball) {
ball->color = WHITE;
score++;
if (ball->prev != NULL && ball->prev->color == WHITE) {
remove_balls(ball->prev);
}
if (ball->next != NULL && ball->next->color == WHITE) {
remove_balls(ball->next);
}
}
// 检测是否有需要消除的球
void check_remove() {
struct Ball* ball = head;
while (ball != NULL) {
if (ball->color != WHITE && check_chain(ball)) {
remove_balls(ball);
ball = tail->next;
while (ball != NULL && ball->color == WHITE) {
ball = ball->next;
}
if (ball == NULL) {
gameover = 1;
break;
}
} else {
ball = ball->next;
}
}
}
// 绘制得分和游戏结束画面
void draw_text() {
char buffer[32];
settextstyle(16, 0, "宋体");
setbkmode(TRANSPARENT);
settextcolor(BLACK);
sprintf(buffer, "得分:%d", score);
outtextxy(WIDTH - 80, 10, buffer);
if (gameover) {
settextstyle(32, 0, "宋体");
settextcolor(RED);
outtextxy(WIDTH / 2 - 100, HEIGHT / 2, "游戏结束");
}
}
// 绘制游戏界面
void draw_game() {
cleardevice();
draw_balls();
draw_launch();
draw_text();
}
// 加载游戏背景和背景音乐
void load_resources() {
loadimage(NULL, "background.jpg", WIDTH, HEIGHT);
if (sound) {
mciSendString("open background.mp3 alias bgmusic", NULL, 0, NULL);
mciSendString("play bgmusic repeat", NULL, 0, NULL);
}
}
// 关闭游戏背景音乐
void close_resources() {
if (sound) {
mciSendString("stop bgmusic", NULL, 0, NULL);
mciSendString("close bgmusic", NULL, 0, NULL);
}
}
// 处理鼠标点击事件
void handle_mouse() {
if (MouseHit()) {
MOUSEMSG msg = GetMouseMsg();
if (msg.uMsg == WM_LBUTTONDOWN) {
int x = msg.x;
int y = msg.y;
if (x >= WIDTH - 50 && x <= WIDTH - 10 &&
y >= HEIGHT - 50 && y <= HEIGHT - 10) {
sound = !sound;
if (sound) {
mciSendString("play bgmusic repeat", NULL, 0, NULL);
} else {
mciSendString("stop bgmusic", NULL, 0, NULL);
}
}
}
}
}
// 主函数
int main() {
initgraph(WIDTH, HEIGHT);
srand(time(NULL));
init_balls();
load_resources();
while (!gameover) {
update_balls();
update_launch();
check_collision();
check_remove();
draw_game();
handle_mouse();
Sleep(30);
}
draw_game();
close_resources();
getch();
closegraph();
return 0;
}
```
在这份代码中,我们使用了双向链表来存储和管理祖玛球链,使用了EasyX图形库来实现游戏界面的绘制和交互,使用了递归算法来检测和消除同色球。同时,我们还使用了多个全局变量来记录游戏状态和参数,使用了多个函数来实现游戏的不同功能。
相关推荐
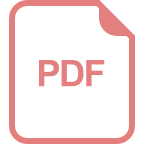
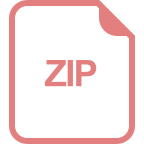
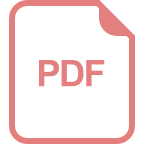














