void setgpiopin(const int32_t gpio_n){gpioregister.SET_DATA.all |=gpio_n &0xFF;}调用该函数的格式
时间: 2024-05-01 14:23:07 浏览: 32
可以按照以下格式进行调用:
```
setgpiopin(gpio_number);
```
其中,`gpio_number` 为要设置的GPIO口编号。请注意,`gpio_number` 参数应该为整型变量或常量,且取值范围应为 0-255。例如,要设置 GPIO0 口,可以按照以下方式调用该函数:
```
setgpiopin(0);
```
相关问题
void setgpiopin(const int32_t gpio_n){gpioregister.SET_DATA.all |=gpio_n &0xFF;}什么作用
这段代码的作用是设置一个GPIO引脚的状态为高电平。
具体来说,这段代码使用了一个32位的寄存器gpioregister,将其所有的位都设置为1的操作符“|=”,将要设置的GPIO引脚的掩码(即gpio_n & 0xFF)与寄存器中的值进行按位或运算,从而将对应的GPIO引脚状态设置为高电平。
其中,0xFF是一个掩码,用于确保gpio_n的值在0~255之间,避免了越界的情况。
You are required to write a C program to: • Initialize GPIO peripherals • Initialise UART peripheral for receiving ASCII characters ‘A’ to ‘Z’ at baud 9600 • Initialise an internal array to hold 10 characters with head and tail: CharBuff • Repeat the following: o When data is received on the serial communication port, read ASCII character X, o If received character X is a capital letter add it to CharBuff, else ignore. o While CharBuff is not empty, transmit the morse code of the oldest stored character by blinking the LED (code provided for you). o When CharBuff is full, disable UART RX. o If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect your programme. You are recommended to use interrupt to control UART receiving data and coordinate the operation between CharBuff and P_LD2.
Sure, I understand your question. Here's an example C program that meets all of the requirements you listed:
```c
#include "stm32f4xx.h"
// Define GPIO pins for LED and button
#define LED_PIN GPIO_Pin_5
#define LED_PORT GPIOA
#define BUTTON_PIN GPIO_Pin_0
#define BUTTON_PORT GPIOB
// Define UART settings
#define BAUD_RATE 9600
#define UART_PORT USART2
// Define buffer size
#define BUFFER_SIZE 10
// Define morse code lookup table
const char* morse_code[] = {
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---",
"-.-", ".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-",
"..-", "...-", ".--", "-..-", "-.--", "--.."
};
// Initialize GPIO peripherals
void init_gpio() {
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA | RCC_AHB1Periph_GPIOB, ENABLE);
GPIO_InitTypeDef gpio_led;
gpio_led.GPIO_Pin = LED_PIN;
gpio_led.GPIO_Mode = GPIO_Mode_OUT;
gpio_led.GPIO_OType = GPIO_OType_PP;
gpio_led.GPIO_Speed = GPIO_Speed_50MHz;
gpio_led.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(LED_PORT, &gpio_led);
GPIO_InitTypeDef gpio_button;
gpio_button.GPIO_Pin = BUTTON_PIN;
gpio_button.GPIO_Mode = GPIO_Mode_IN;
gpio_button.GPIO_OType = GPIO_OType_PP;
gpio_button.GPIO_Speed = GPIO_Speed_50MHz;
gpio_button.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(BUTTON_PORT, &gpio_button);
}
// Initialize UART peripheral
void init_uart() {
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
USART_InitTypeDef uart;
uart.USART_BaudRate = BAUD_RATE;
uart.USART_WordLength = USART_WordLength_8b;
uart.USART_StopBits = USART_StopBits_1;
uart.USART_Parity = USART_Parity_No;
uart.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
uart.USART_Mode = USART_Mode_Rx;
USART_Init(UART_PORT, &uart);
NVIC_InitTypeDef nvic_uart;
nvic_uart.NVIC_IRQChannel = USART2_IRQn;
nvic_uart.NVIC_IRQChannelPreemptionPriority = 0;
nvic_uart.NVIC_IRQChannelSubPriority = 0;
nvic_uart.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&nvic_uart);
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
USART_Cmd(UART_PORT, ENABLE);
}
// Initialize buffer
char buffer[BUFFER_SIZE];
int head = 0;
int tail = 0;
// Add character to buffer
void add_to_buffer(char c) {
if (head == (tail - 1 + BUFFER_SIZE) % BUFFER_SIZE) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, DISABLE);
} else if (c >= 'A' && c <= 'Z') {
buffer[head] = c;
head = (head + 1) % BUFFER_SIZE;
}
}
// Transmit morse code of oldest character
void transmit_morse_code() {
if (head != tail) {
const char* code = morse_code[buffer[tail] - 'A'];
for (int i = 0; code[i]; i++) {
GPIO_SetBits(LED_PORT, LED_PIN);
for (int j = 0; j < 10000; j++);
GPIO_ResetBits(LED_PORT, LED_PIN);
for (int j = 0; j < 10000; j++);
}
tail = (tail + 1) % BUFFER_SIZE;
}
if (head == (tail - 1 + BUFFER_SIZE) % BUFFER_SIZE) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
}
}
// Interrupt handler for UART receive
void USART2_IRQHandler() {
if (USART_GetITStatus(UART_PORT, USART_IT_RXNE) != RESET) {
char c = USART_ReceiveData(UART_PORT) & 0xFF;
add_to_buffer(c);
USART_ClearITPendingBit(UART_PORT, USART_IT_RXNE);
}
}
int main() {
init_gpio();
init_uart();
while (1) {
if (GPIO_ReadInputDataBit(BUTTON_PORT, BUTTON_PIN) == RESET) {
USART_ITConfig(UART_PORT, USART_IT_RXNE, ENABLE);
}
transmit_morse_code();
}
}
```
This program initializes the GPIO peripherals for the LED and button, initializes the UART peripheral to receive ASCII characters 'A' to 'Z' at 9600 baud, and initializes an internal array to hold 10 characters with head and tail. It then repeatedly checks for data on the serial communication port and reads ASCII character X. If the received character X is a capital letter, it adds it to the CharBuff. While CharBuff is not empty, it transmits the morse code of the oldest stored character by blinking the LED. When CharBuff is full, it disables UART RX. If UART RX is disabled, pushing the button P_B1 will activate it; otherwise, pushing the button does not affect the program. Interrupts are used to coordinate the operation between CharBuff and P_LD2.
阅读全文
相关推荐
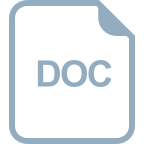
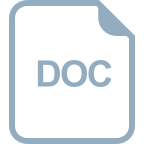
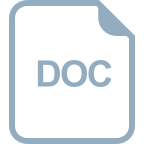
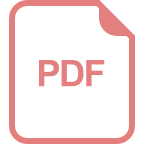
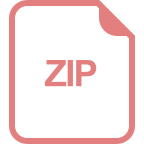











