用java写一个推箱子游戏
时间: 2024-05-29 18:04:14 浏览: 46
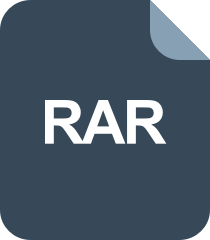
用java编写的推箱子游戏

好的,推箱子游戏是一款比较经典的益智游戏,需要玩家通过移动箱子将它们推到目标位置,我可以为您提供一个简单的Java实现。以下是代码示例:
```java
import java.util.Scanner;
public class PushBoxGame {
// 地图数组,0表示空地,1表示障碍物,2表示目标位置,3表示箱子
private static int[][] map = {
{1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 0, 0, 0, 1},
{1, 0, 2, 0, 3, 0, 1},
{1, 0, 0, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1}
};
// 箱子的初始位置
private static int boxX = 2;
private static int boxY = 4;
// 玩家的初始位置
private static int playerX = 1;
private static int playerY = 1;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
// 打印地图
printMap();
// 判断是否推成功
if (map[boxX][boxY] == 2) {
System.out.println("推箱子成功!");
break;
}
// 获取用户输入
System.out.println("请输入方向(w:上,s:下,a:左,d:右):");
String direction = scanner.next();
// 移动箱子和玩家
switch (direction) {
case "w":
move(-1, 0);
break;
case "s":
move(1, 0);
break;
case "a":
move(0, -1);
break;
case "d":
move(0, 1);
break;
default:
break;
}
}
}
/**
* 移动箱子和玩家
*
* @param dx 箱子和玩家在横向上的偏移量
* @param dy 箱子和玩家在纵向上的偏移量
*/
private static void move(int dx, int dy) {
// 判断箱子和玩家周围是否有障碍物
if (map[boxX + dx][boxY + dy] != 1) {
boxX += dx;
boxY += dy;
playerX += dx;
playerY += dy;
} else if (map[playerX + dx][playerY + dy] != 1) {
playerX += dx;
playerY += dy;
}
}
/**
* 打印地图
*/
private static void printMap() {
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[0].length; j++) {
if (i == boxX && j == boxY) {
System.out.print("3");
} else if (i == playerX && j == playerY) {
System.out.print("0");
} else {
System.out.print(map[i][j]);
}
}
System.out.println();
}
}
}
```
这是一个简单的推箱子游戏实现,您可以根据需要进行修改和完善。
阅读全文
相关推荐
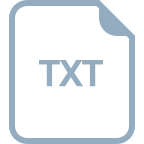










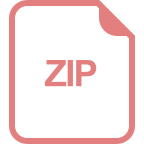
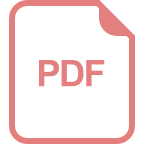
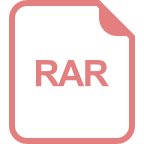


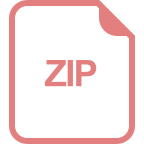