public void OnBackspacePressed() { InputField currentInputField = EventSystem.current.currentSelectedGameObject.GetComponent<InputField>(); if (currentInputField== inputField2) { if (inputField2.text.Length == 0 && UnityEngine.EventSystems.EventSystem.current.currentSelectedGameObject != inputField1.gameObject) { inputField1.text = ""; } } if(currentInputField== inputField3) { if (inputField3.text.Length == 0 && UnityEngine.EventSystems.EventSystem.current.currentSelectedGameObject != inputField2.gameObject) { inputField2.text = ""; } } if(currentInputField== inputField4) { if (inputField4.text.Length == 0 && UnityEngine.EventSystems.EventSystem.current.currentSelectedGameObject != inputField3.gameObject) { inputField3.text = ""; } } }
时间: 2024-02-14 07:33:46 浏览: 132
这段代码的作用是在用户按下退格键时,检查当前选中的输入框是哪一个,并根据该输入框的文本长度和当前选中的游戏对象进行相应的操作。
首先,通过 `EventSystem.current.currentSelectedGameObject` 获取当前被选中的游戏对象,并使用 `GetComponent<InputField>()` 方法获取该游戏对象上的 `InputField` 组件。然后,通过比较当前输入框的引用与 `inputField2`、`inputField3`、`inputField4` 的引用,来确定当前选中的是哪个输入框。
如果当前选中的是 `inputField2`,则在该输入框的文本长度为0并且当前选中的游戏对象不是 `inputField1` 时,将 `inputField1` 的文本设置为空字符串。
如果当前选中的是 `inputField3`,则在该输入框的文本长度为0并且当前选中的游戏对象不是 `inputField2` 时,将 `inputField2` 的文本设置为空字符串。
如果当前选中的是 `inputField4`,则在该输入框的文本长度为0并且当前选中的游戏对象不是 `inputField3` 时,将 `inputField3` 的文本设置为空字符串。
这段代码通常是用于多个输入框之间的联动操作,例如在输入验证码时,用户可以在第一个输入框中输入完毕后,自动跳转到下一个输入框,并在每个输入框中只能输入一个字符,如果用户按下退格键,则自动删除上一个输入框中的字符,以此类推。
相关问题
```csharp using UnityEngine; using TMPro; using UnityEngine.UI; public class ButtonClick : MonoBehaviour { public GameObject prefab; public TMP_Text prefabText; public InputField inputField; public GameObject parentObject; public GameObject[] prefabObject; public void GeneratePrefab() { GameObject newPrefab = Instantiate(prefab); newPrefab.transform.parent = parentObject.transform; TMP_Text newPrefabText = newPrefab.GetComponentInChildren<TMP_Text>(); newPrefabText.text = inputField.text; inputField.onValueChanged.AddListener(delegate { UpdatePrefabText(newPrefabText); }); } public void UpdatePrefabText(TMP_Text newPrefabText) { newPrefabText.text = inputField.text; } }修改这段代码,使得可以追踪到物体不同名字后分别进行生成不同预制体,其他功能不改变。
可以通过修改 GeneratePrefab() 方法来实现追踪不同名字生成不同预制体的功能。可以将输入框中的文本作为预制体的名称,然后在生成预制体时根据名称来选择对应的预制体。
下面是修改后的代码:
```csharp
using UnityEngine;
using TMPro;
using UnityEngine.UI;
public class ButtonClick : MonoBehaviour
{
public TMP_Text prefabText;
public InputField inputField;
public GameObject parentObject;
// 添加一个预制体数组,用于存储不同预制体
public GameObject[] prefabs;
public void GeneratePrefab()
{
string prefabName = inputField.text;
// 根据名称查找对应的预制体
GameObject prefabToGenerate = null;
foreach (GameObject prefab in prefabs)
{
if (prefab.name == prefabName)
{
prefabToGenerate = prefab;
break;
}
}
if (prefabToGenerate != null)
{
GameObject newPrefab = Instantiate(prefabToGenerate);
newPrefab.transform.parent = parentObject.transform;
TMP_Text newPrefabText = newPrefab.GetComponentInChildren<TMP_Text>();
newPrefabText.text = prefabName;
inputField.onValueChanged.AddListener(delegate { UpdatePrefabText(newPrefabText); });
}
else
{
Debug.LogError("Prefab not found with name: " + prefabName);
}
}
public void UpdatePrefabText(TMP_Text newPrefabText)
{
newPrefabText.text = inputField.text;
}
}
```
这里的 prefabs 数组是在 Inspector 窗口中手动指定的,需要将不同预制体拖拽到数组中。在 GeneratePrefab() 方法中,先获取输入框中的文本作为预制体的名称,然后遍历数组查找对应的预制体,最后生成该预制体。如果没有找到对应的预制体,会输出错误信息。
优化这段代码 using System.Collections; using System.Collections.Generic; using UnityEngine; using TMPro; using UnityEngine.UI; public class ButtonClick : MonoBehaviour { public GameObject prefab; public Button button; public TMP_Text prefabText1; public InputField inputField; public GameObject parentObject; public void GeneratePrefab() { // Instantiate prefab parent GameObject newPrefab = Instantiate(prefab); newPrefab.transform.parent = parentObject.transform; // Get TextMeshPro component TMP_Text newPrefabText = newPrefab.GetComponentInChildren<TMP_Text>(); // Update TextMeshPro component's text newPrefabText.text = inputField.text; // Add listener to input field's OnValueChanged event inputField.onValueChanged.AddListener(delegate { UpdatePrefabText(newPrefabText); }); } public void UpdatePrefabText(TMP_Text newPrefabText) { // Update TextMeshPro component's text newPrefabText.text = inputField.text; } }
以下是一些可能有助于代码优化的建议:
1. 在使用 Unity 引擎时,应尽量避免使用 GetComponentInChildren 等方法,因为它们会遍历整个子对象树,从而导致性能问题。相反,你可以将需要访问的组件引用存储在变量中,以便更快地访问它们。
2. 建议使用 Unity 的事件系统来处理按钮点击事件,而不是直接将方法作为按钮的回调。这样可以更容易地管理事件,并且不需要手动设置按钮的回调。
3. 每次调用 GeneratePrefab 方法时,都会添加一个新的委托到 inputField 的 onValueChanged 事件中,这可能会导致内存泄漏。相反,你可以将 UpdatePrefabText 方法作为一个成员变量,并在 Start 方法中添加委托,这样只需要添加一次委托。
4. 建议使用对象池来管理预制体的实例,以便更好地管理内存和性能。
下面是重构后的代码示例:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using TMPro;
using UnityEngine.UI;
public class ButtonClick : MonoBehaviour
{
public GameObject prefab;
public Button button;
public TMP_Text prefabText1;
public InputField inputField;
public GameObject parentObject;
private TMP_Text newPrefabText;
private void Start()
{
// Get TextMeshPro component
newPrefabText = prefab.GetComponentInChildren<TMP_Text>();
// Add listener to input field's OnValueChanged event
inputField.onValueChanged.AddListener(delegate { UpdatePrefabText(); });
}
public void GeneratePrefab()
{
// Instantiate prefab parent
GameObject newPrefab = ObjectPoolManager.Instance.GetObject(prefab);
newPrefab.transform.SetParent(parentObject.transform, false);
// Update TextMeshPro component's text
newPrefabText.text = inputField.text;
}
public void UpdatePrefabText()
{
// Update TextMeshPro component's text
newPrefabText.text = inputField.text;
}
}
注意,这个示例假定你已经实现了对象池管理器。如果没有,请查看这个指南:https://blog.csdn.net/weixin_46202117/article/details/116043594。
阅读全文
相关推荐
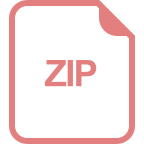
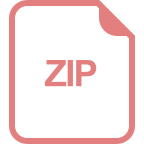











