qt打地鼠小游戏c++
时间: 2023-06-29 14:07:07 浏览: 142
好的,下面是一个简单的打地鼠小游戏的C++代码,使用Qt框架实现:
```c++
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QTimer>
#include <QTime>
#include <QLabel>
#include <QMessageBox>
#include <cstdlib>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
ui->setupUi(this);
setFixedSize(300, 400); // 设置固定的窗口大小
setWindowTitle("打地鼠"); // 设置窗口标题
// 初始化游戏状态
gameStarted = false;
score = 0;
timeLeft = 30;
// 创建计时器
timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(updateTimeLeft()));
// 创建地鼠标签
for (int i = 0; i < 9; i++) {
QLabel *label = new QLabel(this);
label->setPixmap(QPixmap(":/images/mole.png"));
label->setGeometry(20 + (i % 3) * 80, 60 + (i / 3) * 80, 60, 60);
label->hide();
moles.append(label);
}
// 更新游戏面板
updateScore();
updateTimeLeft();
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::updateScore()
{
ui->scoreLabel->setText(QString("得分: %1").arg(score));
}
void MainWindow::updateTimeLeft()
{
if (timeLeft > 0) {
timeLeft--;
ui->timeLabel->setText(QString("剩余时间: %1 秒").arg(timeLeft));
showRandomMole();
} else {
// 游戏结束
timer->stop();
QMessageBox::information(this, "游戏结束", QString("你的得分是 %1 分").arg(score));
gameStarted = false;
score = 0;
timeLeft = 30;
updateScore();
updateTimeLeft();
for (int i = 0; i < 9; i++) {
moles[i]->hide();
}
}
}
void MainWindow::showRandomMole()
{
if (!gameStarted) return;
// 随机选择一个地鼠
int index = qrand() % 9;
QLabel *label = moles[index];
// 如果这个地鼠已经出现了,则重新选择一个地鼠
if (label->isVisible()) {
showRandomMole();
return;
}
// 显示地鼠
label->show();
// 随机选择一个时间,让地鼠消失
int disappearTime = qrand() % 2000 + 1000;
QTimer::singleShot(disappearTime, label, SLOT(hide()));
// 继续显示下一个地鼠
QTimer::singleShot(qrand() % 1000 + 500, this, SLOT(showRandomMole()));
}
void MainWindow::on_startButton_clicked()
{
// 开始游戏
gameStarted = true;
timer->start(1000);
// 隐藏“开始游戏”按钮
ui->startButton->hide();
}
void MainWindow::on_moleLabel_clicked()
{
// 打中了地鼠
if (!gameStarted) return;
score++;
updateScore();
sender()->hide();
}
```
你需要在Qt Creator中新建一个QWidget应用程序工程,然后把上面的代码粘贴到mainwindow.cpp文件中。此外,还需要把一个名为mole.png的图片文件放到工程目录下的images子目录中。运行程序后,点击“开始游戏”按钮即可开始游戏,点击出现的地鼠可以得分。游戏时间为30秒,时间结束后会弹出游戏结束对话框。
相关推荐
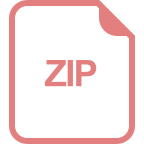














