java贪心算法解决tsp问题
时间: 2023-07-10 18:09:04 浏览: 124
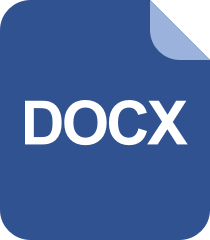
遗传算法解决tsp问题1
TSP问题(旅行商问题)是指一个旅行商要前往n个城市,每个城市只去一次,从某个城市出发,最终回到该城市,问旅行商应该如何选择路径才能使总路程最短。TSP问题是一个经典的NP问题。
贪心算法是求解TSP问题的一种有效方法。具体思路如下:
1. 选择任意一个城市作为起点
2. 从起点开始,选择距离最近的还未访问过的城市作为下一个访问城市,并将该城市标记为已访问
3. 重复步骤2,直到所有城市都被访问
4. 返回最后一个访问城市到起点的距离,作为最终路径长度
Java代码实现:
```java
public class TSP {
public static int tsp(int[][] graph) {
int n = graph.length;
boolean[] visited = new boolean[n];
visited[0] = true;
int current = 0;
int totalDistance = 0;
for (int i = 0; i < n - 1; i++) {
int next = -1;
int minDistance = Integer.MAX_VALUE;
for (int j = 0; j < n; j++) {
if (!visited[j] && graph[current][j] < minDistance) {
minDistance = graph[current][j];
next = j;
}
}
visited[next] = true;
current = next;
totalDistance += minDistance;
}
return totalDistance + graph[current][0]; // 回到起点
}
public static void main(String[] args) {
int[][] graph = {
{0, 4, 3, 6},
{4, 0, 2, 1},
{3, 2, 0, 5},
{6, 1, 5, 0}
};
int shortestDistance = tsp(graph);
System.out.println(shortestDistance);
}
}
```
上述代码中,`graph`为图的邻接矩阵,`tsp`方法返回最短路径长度。
阅读全文
相关推荐
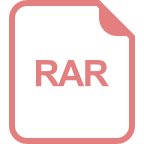















