hadoop实现矩阵相乘的实例
时间: 2023-11-14 07:19:56 浏览: 87
Hadoop实现矩阵相乘的过程可以分为以下几个步骤:
1. 数据准备:将需要相乘的两个矩阵A和B转换为Hadoop分布式文件系统(HDFS)中的数据格式,一般可以使用Java的IO操作将数据从本地文件系统上传到HDFS中。
2. Map阶段:Map任务的输入是矩阵A和B中的一部分,输出是一个键值对,其中键为结果矩阵中的行号,值为该行对应的乘积结果。Map任务的实现需要将矩阵A中某一行的每个元素与矩阵B中对应列的每个元素相乘,将结果累加,最终输出该行的结果。
3. Shuffle阶段:将Map任务的输出按照键值对中的键进行排序和分组,以便后续Reduce任务的处理。
4. Reduce阶段:Reduce任务的输入是Map任务输出的键值对,其中键为结果矩阵中的行号,值为该行对应的所有乘积结果。Reduce任务的实现需要将同一行的所有乘积结果加起来,得到该行的最终结果,最终输出到HDFS文件系统中。
下面是一个简单的Hadoop实现矩阵相乘的示例代码:
```java
public class MatrixMultiplication {
public static class Map extends Mapper<LongWritable, Text, Text, Text> {
private Text key = new Text();
private Text value = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] tokens = value.toString().split(",");
int row = Integer.parseInt(tokens[0]);
int col = Integer.parseInt(tokens[1]);
int val = Integer.parseInt(tokens[2]);
if (tokens[0].equals("A")) {
for (int i = 0; i < N; i++) {
this.key.set(row + "," + i);
this.value.set("A," + col + "," + val);
context.write(this.key, this.value);
}
} else {
for (int i = 0; i < M; i++) {
this.key.set(i + "," + col);
this.value.set("B," + row + "," + val);
context.write(this.key, this.value);
}
}
}
}
public static class Reduce extends Reducer<Text, Text, Text, IntWritable> {
private Text key = new Text();
private IntWritable value = new IntWritable();
public void reduce(Text key, Iterable<Text> values, Context context)
throws IOException, InterruptedException {
int[] A = new int[N];
int[] B = new int[M];
for (Text value : values) {
String[] tokens = value.toString().split(",");
int row = Integer.parseInt(tokens[1]);
int val = Integer.parseInt(tokens[2]);
if (tokens[0].equals("A")) {
A[row] = val;
} else {
B[row] = val;
}
}
int sum = 0;
for (int i = 0; i < N; i++) {
sum += A[i] * B[i];
}
String[] tokens = key.toString().split(",");
int row = Integer.parseInt(tokens[0]);
int col = Integer.parseInt(tokens[1]);
this.key.set(row + "," + col);
this.value.set(sum);
context.write(this.key, this.value);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "matrix multiplication");
job.setJarByClass(MatrixMultiplication.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
job.waitForCompletion(true);
}
}
```
在以上代码中,我们实现了Map和Reduce类来分别实现Map和Reduce任务,Map类将矩阵A和矩阵B中的每个元素转换成键值对,其中键为结果矩阵中的行号,值为该行对应的乘积结果;Reduce类将同一行的所有乘积结果加起来,得到该行的最终结果,最终输出到HDFS文件系统中。
阅读全文
相关推荐
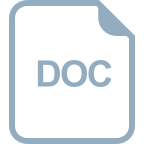
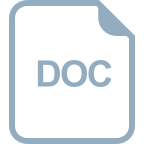
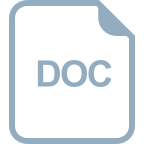

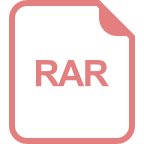



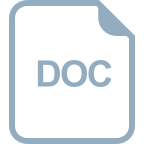
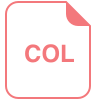
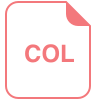
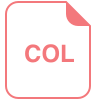
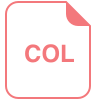
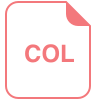
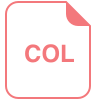
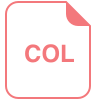
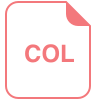
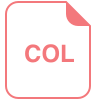