编写一段程序,使其用管道来实现父子进程之间的进程通信。子进程向父进程发 送自己的进程标识符,以及字符串“is sending a message to parent!”。父进程则通过管道读 出子进程发来的消息,将消息显示在屏幕上,然后终止
时间: 2024-11-24 07:41:32 浏览: 25
在Unix/Linux环境中,可以使用`pipe()`函数创建一对管道,然后在父子进程中分别打开这个管道来进行通信。下面是一个简单的Python示例,它展示了如何使用`os`模块和`subprocess`模块来实现这个功能:
```python
import os
# 父进程部分
def parent_process():
# 创建管道
pipe = os.pipe()
# 子进程PID
child_pid = os.fork()
if child_pid == 0: # 子进程
# 关闭不需要的那一端
os.close(pipe[1])
try:
# 向管道写入数据
msg = "is sending a message to parent!"
os.write(pipe[0], msg.encode('utf-8'))
print("Message sent!")
except BrokenPipeError:
pass # 管道关闭,正常情况
finally:
# 子进程结束
os._exit(0) # 使用_exit避免影响父进程的退出状态
else: # 父进程
# 关闭不需要的那一端
os.close(pipe[0])
try:
# 父进程从管道读取数据
msg = os.read(pipe[1], 1024)
print(f"Received from child: {msg.decode('utf-8')}")
except BlockingIOError:
pass # 等待子进程写入
finally:
# 关闭管道并等待子进程结束
os.close(pipe[1])
os.waitpid(child_pid, 0)
if __name__ == "__main__":
parent_process()
```
在这个例子中,父进程首先创建了一个管道,然后创建子进程。子进程接收到管道的写权限,并发送消息到管道,而父进程等待接收并打印消息。完成后,父进程关闭管道并等待子进程结束。
注意,这只是一个基本示例,实际使用时需要处理各种异常和错误情况。另外,Python的标准库中`multiprocessing`模块提供更高级别的进程间通信机制,如`Pipe`,可能更适合复杂的场景。
阅读全文
相关推荐
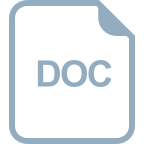
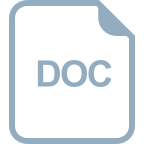
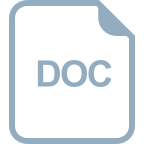





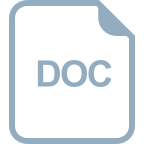
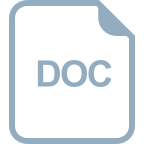
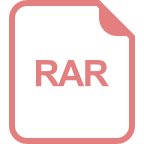
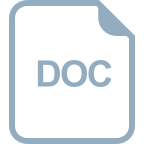
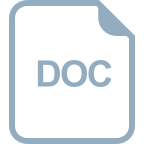
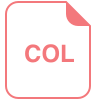
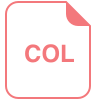
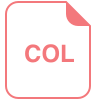
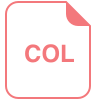
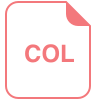
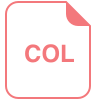