windows系统下,编写一段程序,使其用管道来实现父子进程之间的进程通信。子进程向父进程发送自己的进程标识符,以及字符串“is sending a message to parent!”。父进程则通过管道读出子进程发来的消息,将消息显示在屏幕上,然后终止。
时间: 2023-11-27 15:49:07 浏览: 86
以下是一个示例程序,实现了父子进程之间的进程通信:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
int main()
{
int fd[2]; // 管道文件描述符数组
char buf[100]; // 读取数据的缓冲区
pid_t pid; // 子进程的进程ID
// 创建管道
if (pipe(fd) < 0) {
perror("pipe error");
exit(1);
}
// 创建子进程
if ((pid = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid == 0) { // 子进程
close(fd[0]); // 关闭读取端
int pid = getpid();
char msg[] = "is sending a message to parent!";
write(fd[1], &pid, sizeof(pid)); // 发送进程ID
write(fd[1], msg, sizeof(msg)); // 发送消息
close(fd[1]); // 关闭写入端
exit(0);
} else { // 父进程
close(fd[1]); // 关闭写入端
int pid;
read(fd[0], &pid, sizeof(pid)); // 读取进程ID
read(fd[0], buf, sizeof(buf)); // 读取消息
printf("Child process %d %s\n", pid, buf);
close(fd[0]); // 关闭读取端
}
return 0;
}
```
该程序中,首先创建了一个管道,然后通过 fork() 函数创建了一个子进程。子进程向父进程发送自己的进程标识符和消息,父进程通过管道读取子进程发送的消息,并将消息显示在屏幕上,最后终止程序。在程序中,管道的读取端和写入端分别用数组中的第 0 和第 1 个元素表示。为了避免出现死锁的情况,需要在父进程和子进程中分别关闭管道的对应端口。
相关推荐
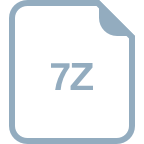
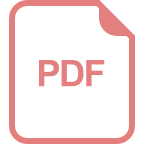
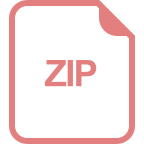














