编写一段程序,使用管道来实现父子进程之间的进程通信。
时间: 2024-05-09 19:18:00 浏览: 149
可以的,以下是一个使用管道实现父子进程之间通信的示例程序:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define BUFFER_SIZE 1024
int main()
{
int fd[2];
pid_t pid;
char buffer[BUFFER_SIZE];
if (pipe(fd) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
pid = fork();
if (pid < 0) {
perror("fork error");
exit(EXIT_FAILURE);
}
if (pid > 0) { // parent process
close(fd[0]); // close read end of the pipe
printf("Parent process sending message...\n");
write(fd[1], "Hello, child process!", strlen("Hello, child process!"));
close(fd[1]); // close write end of the pipe
} else { // child process
close(fd[1]); // close write end of the pipe
printf("Child process receiving message...\n");
read(fd[0], buffer, BUFFER_SIZE);
printf("Message received: %s\n", buffer);
close(fd[0]); // close read end of the pipe
}
return 0;
}
```
这个程序创建了一个管道,并使用 `fork()` 函数创建了一个子进程。在父进程中,它向管道写入一条消息,然后关闭管道的写端。在子进程中,它从管道读取消息并将其打印到屏幕上,然后关闭管道的读端。
注意,父子进程之间的管道通信是单向的,因此需要创建两个管道分别用于父进程向子进程发送消息和子进程向父进程发送消息。在本示例中,我们只使用了一个管道,因为我们只需要在父进程向子进程发送一条消息。
阅读全文
相关推荐
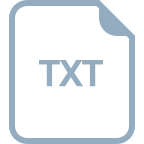












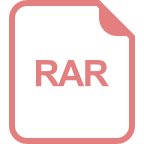
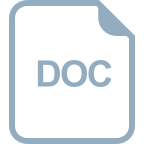
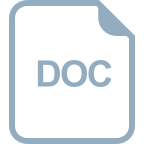
