编写一个Java程序,设计一个汽车类Vehicle,包含的属性有车轮个 数wheels和车重weight。小车类Car是Vehicle的子类,其中包含 的属性有载人数loader。卡车类Truck是Car类的子类,其中包含 的属性有载重量payload。 (1)每个类都有构造方法进行属性初始化; (2)每个类都输出相关数据信息的成员方法; (3)使用Test类中的main方法实例化各类,并输出相关信息。
时间: 2023-06-20 14:08:02 浏览: 710
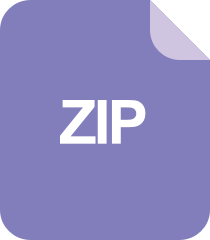
java代码-请定义一个交通工具(Vehicle)的类,其中有: 属性:速度(speed),体积(size)等等 方 法: 移 动(move()) , 设置 速 度(setSpeed(int speed)) , 加速 speedUp(), 减速 speedDown()等等. 最后在测试类 Vehicle 中的 main()中实例化一个交通工具对象,并通过方法给它初始化 speed,size 的值,并且通过打印出来。另外,调用加速,减速的方法对速度进行改变。
下面是Java程序的实现:
```
class Vehicle {
private int wheels;
private double weight;
public Vehicle(int wheels, double weight) {
this.wheels = wheels;
this.weight = weight;
}
public int getWheels() {
return wheels;
}
public double getWeight() {
return weight;
}
public void printInfo() {
System.out.println("Wheels: " + wheels + ", Weight: " + weight);
}
}
class Car extends Vehicle {
private int loader;
public Car(int wheels, double weight, int loader) {
super(wheels, weight);
this.loader = loader;
}
public int getLoader() {
return loader;
}
public void printInfo() {
super.printInfo();
System.out.println("Loader: " + loader);
}
}
class Truck extends Car {
private double payload;
public Truck(int wheels, double weight, int loader, double payload) {
super(wheels, weight, loader);
this.payload = payload;
}
public double getPayload() {
return payload;
}
public void printInfo() {
super.printInfo();
System.out.println("Payload: " + payload);
}
}
public class Test {
public static void main(String[] args) {
Vehicle v = new Vehicle(4, 1000.0);
Car c = new Car(4, 1200.0, 5);
Truck t = new Truck(6, 5000.0, 2, 10000.0);
v.printInfo();
c.printInfo();
t.printInfo();
}
}
```
在Test类中,我们实例化了一个Vehicle对象v、一个Car对象c和一个Truck对象t,并分别调用它们的printInfo方法输出相关信息。其中,Car和Truck类继承了Vehicle类的属性和方法,并增加了自己的属性和方法。在输出信息时,我们先调用父类的printInfo方法,再输出自己的属性信息。
阅读全文
相关推荐
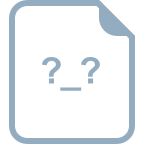
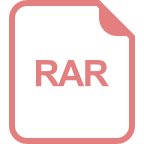














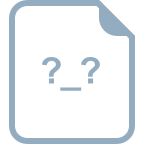