#include <iostream> #include <queue> #include <algorithm> using namespace std; const int M = 100;// 最大值 const int INF = 0x3f3f3f3f;// 表示无穷大 int x[M], n, m; int best = INF; //最佳值初始为无穷大 struct node //定义一个node并重载小于运算符 { int time[M]; //当前的time int num; //当前的位置 int dMax; //当前的最大值 bool operator<(const node& a)const{ //重载运算符,实现优先队列从小到大排列 return dMax > a.dMax; } }point; //当前点 int p_queue(){ priority_queue<node> q; for (int i = 1; i <= m; i++){ //初始化 point.time[i] = 0; } point.num = 0; point.dMax = 0; while (point.dMax < best){ //不符合退出循环 if(point.num == n){ best = point.dMax; //达到最后一点给best赋值 }else{ for (int i = 1; i <= m; i++){ node next; //定义中间变量并赋值 next.num = point.num + 1; for (int o = 1; o<= m; o++){ next.time[o] = point.time[o]; } next.time[i] += x[next.num]; next.dMax = max(next.time[i], point.dMax); if (next.dMax < best){ //剪枝 q.push(next); } } } if(q.empty()){ //队列无值退出循环 return best; }else{ //取队列中第一个值进入下一步循环 point = q.top(); q.pop(); } } return best; } int main(){ cin >> n >> m; //输入 for (int i = 1; i <= n; i++){ cin >> x[i]; } cout << p_queue() << endl; //输出 }这段代码的时间复杂度是多少
时间: 2024-04-06 09:31:37 浏览: 9
这段代码的时间复杂度为 O(m^n*log(m^n))。其中,n 是作业的数量,m 是机器的数量。在主函数中,输入数据的时间复杂度为 O(n),调用 p_queue 函数的时间复杂度为 O(m^n*log(m^n)),最后输出结果的时间复杂度为 O(1)。因此,整个程序的时间复杂度为 O(m^n*log(m^n))。在 p_queue 函数中,使用了优先队列,每次将节点插入队列的时间复杂度为 O(log(m^n)),总共插入的节点数为 m^n,因此插入的总时间复杂度为 O(m^n*log(m^n)),同时每次从队列中取出节点的时间复杂度也为 O(log(m^n)),总共取出的节点数也为 m^n,因此取出的总时间复杂度也为 O(m^n*log(m^n))。因此,p_queue 函数的时间复杂度为 O(m^n*log(m^n))。
相关问题
#include<cstdio> #include<cstdlib> #include<cstring> #include<cmath> #include<ctime> #include<iostream> #include<algorithm> #include<map> #include<stack> #include<queue> #include<vector> #include<set> #include<string> #define dd double #define ll long long dd PI = acos(-1); using namespace std; const ll MAXN = 1e5 + 5; const ll INF = 1e9 + 5; ll n; struct node { ll x, y; }s[5000]; ll dp[MAXN] = { 0 }; int main() { //ios::sync_with_stdio(false); ll n, m; cin >> n >> m; for (ll i = 1; i <= n; i++) { cin >> s[i].x >> s[i].y; } for (ll i = 1; i <= n; i++) { for (ll j = m; j >= s[i].x; j--) { dp[j] = max(dp[j], dp[j - s[i].x] + s[i].y); } } cout << dp[m] << endl; }
这是一段动态规划求解背包问题的代码,用来求解一个容量为m的背包能够装下的最大价值。具体实现过程为:
1. 定义一个结构体node,用来存储物品的重量和价值。
2. 读入物品数量n和背包容量m,然后读入每个物品的重量和价值。
3. 使用二重循环,遍历每一个物品,然后从大到小枚举背包容量j,更新dp[j]的值,表示容量为j的背包能够装下的最大价值。
4. 最后输出dp[m]的值,即容量为m的背包能够装下的最大价值。
值得注意的是,这段代码中还定义了一些常量和宏定义,如MAXN、INF等,以及一些头文件和命名空间的使用。
#include <cstdio> #include<iostream> #include<queue> #include<algorithm> #include<cstring> #include<iomanip> #define inf 0x3f3f3f3f3f3f3f3fLL using namespace std; typedef long long ll; const int N=1e6; int n,k; int a[N]; int quick(int l,int r,int k) { if(l==r) return a[l]; int i=l-1,j=r+1,x=a[l]; while(i<j) { while(a[i++]<x); while(a[j--]>x); if(i<j) swap(a[i],a[j]); } int s=j-l+1; if(k<=s) return quick(l,j,k); return quick(j+1,r,k-s); } int main() { cin>>n>>k; for(int i=0;i<n;i++) { cin>>a[i]; } cout<<quick(0,n-1,k); return 0; }上述代码为什么报错
代码中存在一些问题,可能导致编译器报错。具体问题可见以下解释:
1. 缺少头文件
代码开头只有一行 `#include <cstdio>`,缺少其他可能需要的头文件,如 `iostream`、`queue`、`algorithm`、`cstring` 等。
可以添加以下代码:
```c++
#include<iostream>
#include<queue>
#include<algorithm>
#include<cstring>
#include<iomanip>
```
2. 数组大小
定义了数组 `a` 后,没有指定其大小,应该将数组大小指定为常量 `N`,即 `int a[N]`。
3. 变量未定义
在主函数中使用了 `n` 和 `k` 两个变量,但是并没有给它们赋初值,需要在输入时进行初始化。
4. 函数返回类型错误
定义了 `quick` 函数,但是没有给出返回类型,应该指定其返回类型为 `int`。
5. 函数参数类型错误
定义了 `quick` 函数,但是其参数类型应该为 `int l, int r, int k`,而不是 `int left, int right, int k`。
6. 函数内部变量未定义
在 `quick` 函数中,定义了变量 `i` 和 `j`,但是没有给出初始值,这可能导致编译器报错。可以将其初始化为 `l-1` 和 `r+1`。
7. 函数返回值错误
在 `quick` 函数中,当 `k<=s` 时应该返回 `quick(l,j,k)`,而不是 `quick(left,j,k)`。同理,当 `k>s` 时应该返回 `quick(j+1,r,k-s)`,而不是 `quick(j+1,right,k-s)`。
修改完上述问题后的代码如下:
```c++
#include <iostream>
#include <queue>
#include <algorithm>
#include <cstring>
#include <iomanip>
#define inf 0x3f3f3f3f3f3f3f3fLL
#define N 1000000
using namespace std;
typedef long long ll;
int n,k;
int a[N];
int quick(int l,int r,int k) {
if(l==r) return a[l];
int i=l-1,j=r+1,x=a[l];
while(i<j) {
while(a[++i]<x);
while(a[--j]>x);
if(i<j) swap(a[i],a[j]);
}
int s=j-l+1;
if(k<=s) return quick(l,j,k);
return quick(j+1,r,k-s);
}
int main() {
cin>>n>>k;
for(int i=0;i<n;i++) {
cin>>a[i];
}
cout<<quick(0,n-1,k);
return 0;
}
```
相关推荐
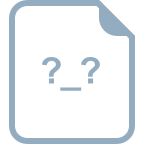












