编写一个程序,两个进程使用消息队列进行通信,传递tmp.txt文本的内容。
时间: 2024-02-25 07:58:12 浏览: 62
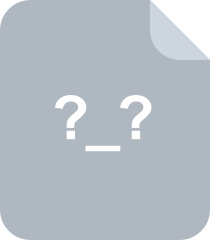
消息队列程序,进程间通信。
以下是一个使用消息队列实现进程间通信,传递文件内容的简单示例程序:
发送进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#include <sys/types.h>
#define MSG_TYPE 1
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main(int argc, char *argv[]) {
int msgid;
struct msgbuf buf;
key_t key;
if (argc < 2) {
printf("Usage: %s <filename>\n", argv[0]);
exit(1);
}
FILE *fp = fopen(argv[1], "r");
if (fp == NULL) {
printf("Failed to open file: %s\n", argv[1]);
exit(1);
}
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid < 0) {
printf("Failed to create message queue\n");
exit(1);
}
// 读取文件内容并发送消息
buf.mtype = MSG_TYPE;
while (fgets(buf.mtext, MSG_SIZE, fp)) {
msgsnd(msgid, &buf, sizeof(buf.mtext), 0);
}
// 发送结束标志
buf.mtype = MSG_TYPE + 1;
strcpy(buf.mtext, "EOF");
msgsnd(msgid, &buf, sizeof(buf.mtext), 0);
fclose(fp);
return 0;
}
```
接收进程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#include <sys/types.h>
#define MSG_TYPE 1
#define MSG_SIZE 1024
struct msgbuf {
long mtype;
char mtext[MSG_SIZE];
};
int main(int argc, char *argv[]) {
int msgid;
struct msgbuf buf;
key_t key;
// 创建消息队列
key = ftok(".", 'a');
msgid = msgget(key, IPC_CREAT | 0666);
if (msgid < 0) {
printf("Failed to create message queue\n");
exit(1);
}
// 接收消息并输出
while (1) {
msgrcv(msgid, &buf, sizeof(buf.mtext), MSG_TYPE, 0);
if (strcmp(buf.mtext, "EOF") == 0) {
break;
}
printf("%s", buf.mtext);
}
// 删除消息队列
msgctl(msgid, IPC_RMID, NULL);
return 0;
}
```
以上程序通过创建一个消息队列,发送进程将文件内容按行发送到消息队列中,接收进程从消息队列中读取数据并输出。需要注意的是,此处为了简化示例程序,没有进行错误处理和并发控制。
阅读全文
相关推荐

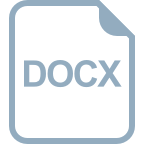
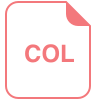
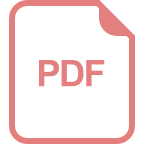
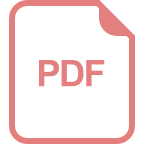
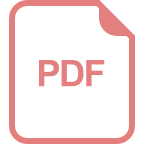
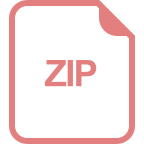
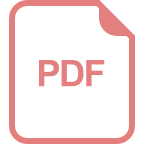