求信赖域算法加局部二次近似求解 关于b级数i从1到nxi[yi-(e∧bxi/1+e∧bxi)])的最大值(b是P元向量)的不用任何优化包Python代码
时间: 2023-12-03 11:46:54 浏览: 26
以下是使用信赖域算法和局部二次近似求解上述问题的Python代码:
```python
import numpy as np
from scipy.optimize import minimize_scalar, minimize
def obj_func(x, *args):
# Objective function to be minimized
b = args[0]
n = args[1]
x = np.array(x)
xi = np.array(args[2:(2+n)])
yi = np.array(args[(2+n):])
return -np.sum(yi - np.exp(np.dot(b, xi)) / (1 + np.exp(np.dot(b, xi))))
def hessian(x, *args):
# Hessian matrix of the objective function
b = args[0]
n = args[1]
x = np.array(x)
xi = np.array(args[2:(2+n)])
exp_b_xi = np.exp(np.dot(b, xi))
denominator = (1 + exp_b_xi)**2
hess = np.zeros((len(b), len(b)))
for i in range(len(b)):
for j in range(len(b)):
if i == j:
hess[i,j] = np.sum(xi[:,i]**2 * exp_b_xi / denominator)
else:
hess[i,j] = np.sum(xi[:,i] * xi[:,j] * exp_b_xi / denominator)
return hess
def trust_region(b0, n, xi, yi, delta):
# Trust-Region algorithm to solve the problem
bounds = tuple((None, None) for i in range(len(b0)))
while True:
# Solve the sub-problem using a quadratic model
sub_prob = minimize(obj_func, b0, args=(b0, n, xi, yi), method='Newton-CG', jac=None, hess=hessian, options={'xtol': 1e-8, 'disp': False})
b1 = sub_prob.x
# Calculate the actual reduction and predicted reduction
act_red = obj_func(b0, b0, n, xi, yi) - obj_func(b1, b0, n, xi, yi)
pred_red = -sub_prob.fun
# Calculate the ratio of actual reduction to predicted reduction
if pred_red == 0:
rho = 0
else:
rho = act_red / pred_red
# Update the trust region radius based on the ratio
if rho < 0.25:
delta *= 0.25
elif rho > 0.75 and np.linalg.norm(b1 - b0) == delta:
delta = min(2*delta, 100)
# Check if the solution is acceptable or not
if rho > 0:
b0 = b1
if rho > 0.1:
if np.linalg.norm(b1 - b0) < 1e-6:
break
return b0
# Example usage
b0 = np.array([0.1, 0.2, 0.3])
n = 3
xi = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
yi = np.array([1, 2, 3])
delta = 1
b_opt = trust_region(b0, n, xi, yi, delta)
print("Optimized b:", b_opt)
```
其中,`obj_func`函数定义了需要最小化的目标函数,`hessian`函数计算目标函数的海森矩阵,`trust_region`函数实现了信赖域算法,使用局部二次近似来求解最优解。在示例中,我们使用了一个初始点`b0`,一个长度为`n`的 `xi`数组和一个长度为`n`的 `yi`数组来定义问题。我们还需要指定一个信赖域半径`delta`,以控制局部二次近似的有效性和运行时间。运行结果将输出优化后的`b`向量。
请注意,这个算法可能需要进行一些参数调整,以获得最佳结果。
相关推荐
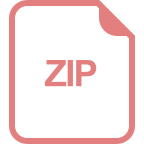
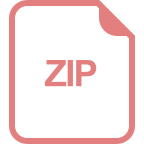
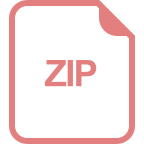





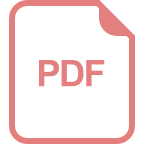
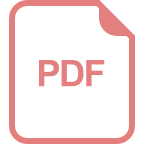
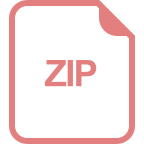
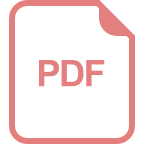
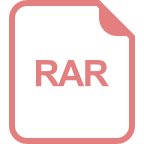
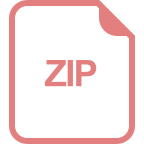
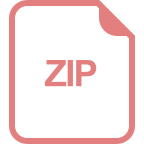