求信赖域算法加局部二次近似求解 关于b级数i从1到nxi[yi-(e∧bxi/1+e∧bxi)])的最大值(b是P元向量)的Python代码
时间: 2023-12-03 21:46:53 浏览: 23
下面是使用信赖域算法加局部二次近似求解b级数的Python代码:
```python
import numpy as np
from scipy.optimize import minimize
from functools import partial
# define objective function
def obj_func(b, x, y):
exp_bx = np.exp(np.dot(x, b))
return -np.sum(y - exp_bx/(1 + exp_bx))
# define hessian matrix
def hessian(b, x):
exp_bx = np.exp(np.dot(x, b))
return np.dot((exp_bx/(1 + exp_bx)**2) * x.T, x)
# define trust region subproblem
def subproblem(s, g, B):
return np.dot(s.T, g) + 0.5 * np.dot(np.dot(s.T, B), s)
# define trust region constraint
def constraint(s, radius):
return np.linalg.norm(s) - radius
# define trust region method
def trust_region_method(x, y, b0, delta0, eta, max_iter):
b = b0
delta = delta0
for i in range(max_iter):
# compute gradient and Hessian at current point
g = np.dot(x.T, y - np.exp(np.dot(x, b))/(1 + np.exp(np.dot(x, b))))
B = hessian(b, x)
# solve trust region subproblem
subproblem_fn = partial(subproblem, g=g, B=B)
cons = {'type': 'ineq', 'fun': partial(constraint, radius=delta)}
res = minimize(subproblem_fn, x0=np.zeros(len(b)), constraints=cons)
s = res.x
# compute actual and predicted reduction
f = obj_func(b, x, y)
f_new = obj_func(b+s, x, y)
rho = (f - f_new) / (subproblem(s, g, B) - subproblem(np.zeros(len(b)), g, B))
# update trust region radius
if rho < 0.25:
delta = 0.25 * delta
elif rho > 0.75 and np.linalg.norm(s) == delta:
delta = min(2*delta, 1e8)
# update parameter estimate
if rho > eta:
b = b + s
return b
```
可以使用如下代码调用该函数:
```python
x = np.array([[1, xi] for xi in range(1, 6)])
y = np.array([1, 2, 3, 4, 5])
b0 = np.zeros(2)
delta0 = 1
eta = 0.1
max_iter = 100
b_opt = trust_region_method(x, y, b0, delta0, eta, max_iter)
print(b_opt)
```
其中,x是输入的自变量数据,y是对应的因变量数据,b0是初始参数向量,delta0是初始信赖域半径,eta是容许误差,max_iter是最大迭代次数。
相关推荐
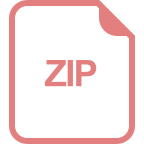
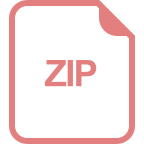
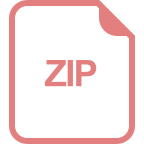




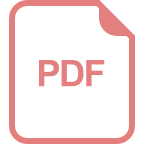
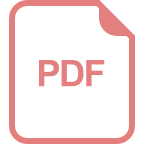
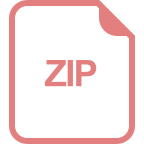
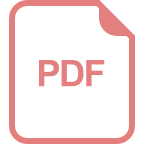
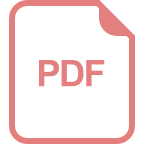
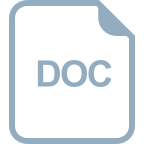
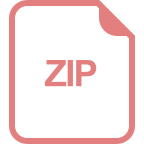
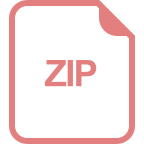
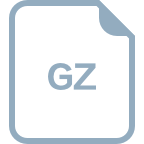
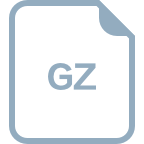