用C语言实现将512*512*100大小的三维数组转换成为512*5120*10大小的三维数组,并打印输出转换后的三维数组
时间: 2024-09-09 14:06:34 浏览: 42
在C语言中,可以使用指针和内存操作来实现这种维度调整。假设原始数组`orig_data`是一个`unsigned char`类型的512x512x100的三维数组,而目标数组`new_data`应是512x5120x10。首先,我们需要计算新的数据区域的起始地址,然后复制原数组的数据到新数组。这里是一种简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define ORIG_WIDTH 512
#define ORIG_DEPTH 100
#define NEW_WIDTH 512
#define NEW_DEPTH 5120
// 假设原始三维数组已经初始化好
unsigned char *orig_data;
unsigned char *new_data;
void resize_array() {
// 计算新的数据区域的起始地址
new_data = (unsigned char *)malloc((NEW_WIDTH * NEW_DEPTH * 10) * sizeof(unsigned char));
if (new_data == NULL) {
printf("Memory allocation failed!\n");
return;
}
// 将原数组数据复制到新数组
for (int i = 0; i < NEW_WIDTH * NEW_DEPTH * 10; i++) {
new_data[i] = orig_data[i * ORIG_WIDTH * ORIG_DEPTH / 10]; // 缩小了10倍
}
// 打印新数组的内容(这里省略实际打印部分,因为通常不会直接打印这么大的数据)
// ... (添加适当的打印函数)
// 清理内存
free(orig_data);
orig_data = NULL;
}
int main() {
// 初始化或获取原始数组
orig_data = ... // 初始化或动态分配内存给原始数组
// 调整并打印新数组
resize_array();
return 0;
}
```
阅读全文
相关推荐
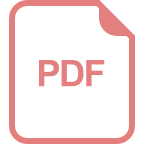
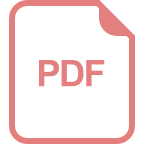
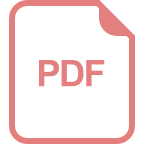









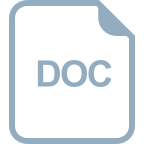





