用java定义一个类Dog,要求具有String型的name、int型的age两个private访问控制的成员变量,同时提供两个public的成员变量的访问方法getName()、getAge(),两个成员变量设置方法setName(String newName)、setAge(int newAge)
时间: 2023-06-13 20:06:34 浏览: 103
以下是定义一个类Dog的Java代码:
```
public class Dog {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String newName) {
name = newName;
}
public int getAge() {
return age;
}
public void setAge(int newAge) {
age = newAge;
}
}
```
以上代码定义了一个名为Dog的类,它具有两个私有成员变量name和age。通过公共访问方法getName()和getAge(),可以获取这两个成员变量的值;通过公共访问方法setName()和setAge(),可以设置这两个成员变量的值。
相关问题
已知有如下Animal抽象类,请编写其子类Dog类与Cat类,另外再编写一个生产动物的Factory工厂类,具体要求如下。 已有的Animal抽象类定义: abstract class Animal{ private String name; //名字 private int age; //年龄 public abstract void info(); //返回动物信息 public abstract void speak(); //动物叫 public Animal(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } } 需要你编写的Dog子类: 增加violence(凶猛程度)属性(int型),重写info和speak方法 info方法输出Dog的name、age和violence属性,输出格式样例为:该狗的名字是Mike,年龄是2岁,凶猛程度是78度 (注意:输出结果中没有空格,逗号为英文标点符号) speak方法输出Dog 的叫声,输出格式样例为:旺旺 需要你编写的Cat子类: 增加mousingAbility(捕鼠能力)属性(int型),重写info和speak方法 info方法输出Cat的name、age和mousingAbility属性,输出格式样例为:该猫的名字是Kitty,年龄是4岁,捕鼠能力是88分 (注意:输出结果中没有空格,逗号为英文标点符号) speak方法输出Cat 的叫声,输出格式样例为:喵喵 需要你编写的Factory工厂类: Animal getAnimalByType(int type,String name, int age, int ownAttribute):根据传入的子类类型和属性值,返回一个Animal类型的上转型对象,type为1代表狗类,type为2代表猫类
。如果传入的type值不为1或2,则返回null。
Factory类的代码如下:
public class Factory {
public Animal getAnimalByType(int type, String name, int age, int ownAttribute) {
if (type == 1) {
return new Dog(name, age, ownAttribute);
} else if (type == 2) {
return new Cat(name, age, ownAttribute);
} else {
return null;
}
}
}
Dog子类的代码如下:
public class Dog extends Animal {
private int violence;
public Dog(String name, int age, int violence) {
super(name, age);
this.violence = violence;
}
public void info() {
System.out.print("该狗的名字是" + getName() + ",年龄是" + getAge() + ",凶猛程度是" + violence + "度");
}
public void speak() {
System.out.print("旺旺");
}
}
Cat子类的代码如下:
public class Cat extends Animal {
private int mousingAbility;
public Cat(String name, int age, int mousingAbility) {
super(name, age);
this.mousingAbility = mousingAbility;
}
public void info() {
System.out.print("该猫的名字是" + getName() + ",年龄是" + getAge() + ",捕鼠能力是" + mousingAbility + "分");
}
public void speak() {
System.out.print("喵喵");
}
}
以上就是根据题目要求写出的Animal抽象类、Dog子类、Cat子类以及Factory工厂类的代码。通过Factory类获取Animal对象时,传入的type参数可以用一个变量来接收用户的输入,然后根据实际情况调用Factory类的getAnimalByType方法来获取相应的Animal对象。
#include <iostream> using namespace std; class Animal { private: int age; public: void setAge(int a) { age = a; } int getAge() { return age; } }; class Dog : public Animal { private: string name; public: void setName(string n) { name = n; } string getName() { return name; } }; class Dog_hair : public Animal { private: string hair; public: void sethair(string m) { hair = m; } string gethair() { return hair; } }; int main() { Dog myDog; myDog.setAge(3); myDog.setName("Lucky"); myDog.sethair("roe"); // 父类Animal访问子类Dog的name变量 // cout << "My dog's name is " << static_cast<Dog*>(&myDog)->getName() << endl; cout << "My dog's name is " << myDog.getName() << endl; // 父类Animal访问子类Dog的age变量 cout << "My dog's age is " << myDog.getAge() << endl; return 0; }父类调不到myDog.sethair("roe"); 该咋样解决
在这段代码中,父类 Animal 没有访问子类 Dog_hair 的 sethair 函数的权限,因为这个函数只在子类 Dog_hair 中定义了而不在父类 Animal 中。如果你想要在父类 Animal 中访问子类 Dog_hair 的 sethair 函数,你可以在父类 Animal 中定义一个虚函数,然后在子类 Dog_hair 中重写这个虚函数。
例如,你可以在 Animal 类中定义一个虚函数:
```c++
class Animal {
private:
int age;
public:
void setAge(int a) {
age = a;
}
int getAge() {
return age;
}
virtual void sethair(string m) {}
};
```
在 Dog_hair 类中重写这个虚函数:
```c++
class Dog_hair : public Animal {
private:
string hair;
public:
void sethair(string m) {
hair = m;
}
string gethair() {
return hair;
}
};
```
然后你就可以在主函数中通过父类 Animal 的指针访问子类 Dog_hair 的 sethair 函数了:
```c++
Animal* myDog = new Dog_hair;
myDog->setAge(3);
myDog->sethair("roe");
cout << "My dog's hair is " << static_cast<Dog_hair*>(myDog)->gethair() << endl;
```
注意,这里需要使用动态分配的指针,并且需要使用 static_cast 将其转换为 Dog_hair 类型指针,以便访问子类的 gethair 函数。
阅读全文
相关推荐
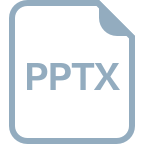
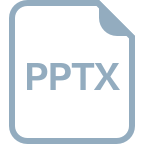













