java写一个登陆实现
时间: 2023-05-11 18:07:38 浏览: 118
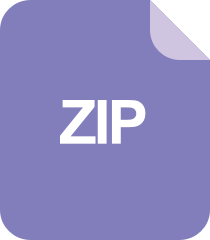
java实现登录操作
可以使用 Java Servlet 和 JSP 技术实现一个简单的登录系统。首先,需要创建一个登录页面,用户可以在该页面输入用户名和密码。然后,将用户输入的用户名和密码发送到服务器端进行验证。如果验证通过,就将用户重定向到主页;否则,就返回登录页面并显示错误消息。以下是一个简单的登录系统的示例代码:
login.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="login" method="post">
<label>Username:</label>
<input type="text" name="username"><br>
<label>Password:</label>
<input type="password" name="password"><br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
LoginServlet.java:
```
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if ("admin".equals(username) && "password".equals(password)) {
response.sendRedirect("home.jsp");
} else {
request.setAttribute("error", "Invalid username or password");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
}
}
```
home.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Home</title>
</head>
<body>
<h1>Welcome!</h1>
</body>
</html>
```
在这个示例中,我们使用了 Java Servlet 和 JSP 技术实现了一个简单的登录系统。用户可以在 login.jsp 页面输入用户名和密码,然后将这些信息发送到 LoginServlet 进行验证。如果验证通过,就将用户重定向到 home.jsp 页面;否则,就返回 login.jsp 页面并显示错误消息。
阅读全文
相关推荐
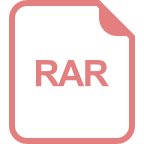
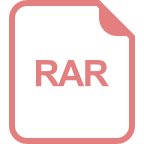
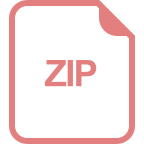
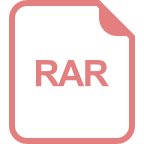
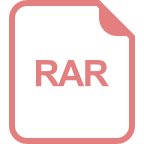
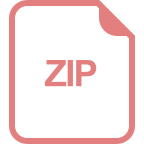
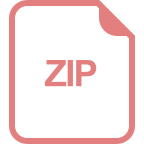
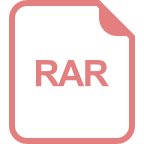
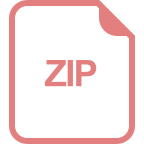
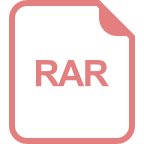
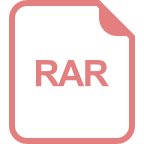
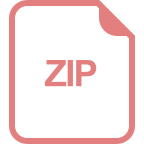
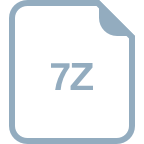
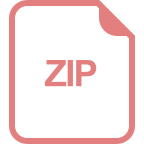