路径规划算法stm32代码
时间: 2023-07-26 18:24:53 浏览: 185
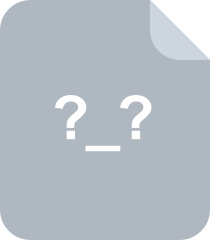
stm32的代码
路径规划算法有很多种,比如A*算法、Dijkstra算法等。在STM32中实现路径规划需要考虑到算法的复杂度和实时性等问题。以下是一个A*算法的简单示例代码:
```c
#define MAP_WIDTH 10
#define MAP_HEIGHT 10
// 定义地图
int map[MAP_HEIGHT][MAP_WIDTH] = {
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 1, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 1, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 1, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 1, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
};
// 定义节点类型
typedef struct {
int x;
int y;
int g;
int h;
int f;
int parent_x;
int parent_y;
} node_t;
// 定义开放列表和关闭列表
node_t open_list[MAP_WIDTH * MAP_HEIGHT];
node_t close_list[MAP_WIDTH * MAP_HEIGHT];
int open_list_count = 0;
int close_list_count = 0;
// 计算两个节点之间的距离
int distance(int x1, int y1, int x2, int y2) {
int dx = abs(x1 - x2);
int dy = abs(y1 - y2);
return dx + dy;
}
// 判断节点是否在开放列表中
int in_open_list(int x, int y) {
int i;
for (i = 0; i < open_list_count; i++) {
if (open_list[i].x == x && open_list[i].y == y) {
return 1;
}
}
return 0;
}
// 判断节点是否在关闭列表中
int in_close_list(int x, int y) {
int i;
for (i = 0; i < close_list_count; i++) {
if (close_list[i].x == x && close_list[i].y == y) {
return 1;
}
}
return 0;
}
// 将节点加入到开放列表中
void add_to_open_list(int x, int y, int g, int h, int parent_x, int parent_y) {
int i;
for (i = 0; i < open_list_count; i++) {
if (open_list[i].x == x && open_list[i].y == y) {
if (open_list[i].f > g + h) {
open_list[i].g = g;
open_list[i].h = h;
open_list[i].f = g + h;
open_list[i].parent_x = parent_x;
open_list[i].parent_y = parent_y;
}
return;
}
}
open_list[open_list_count].x = x;
open_list[open_list_count].y = y;
open_list[open_list_count].g = g;
open_list[open_list_count].h = h;
open_list[open_list_count].f = g + h;
open_list[open_list_count].parent_x = parent_x;
open_list[open_list_count].parent_y = parent_y;
open_list_count++;
}
// 获取开放列表中的最小节点
int get_min_f_node() {
int i;
int min_f = INT_MAX;
int min_index = -1;
for (i = 0; i < open_list_count; i++) {
if (open_list[i].f < min_f) {
min_f = open_list[i].f;
min_index = i;
}
}
return min_index;
}
// 寻找路径
int find_path(int start_x, int start_y, int end_x, int end_y) {
int i;
int current_x, current_y, new_x, new_y;
int g, h, f;
node_t current_node;
node_t new_node;
open_list_count = 0;
close_list_count = 0;
add_to_open_list(start_x, start_y, 0, distance(start_x, start_y, end_x, end_y), -1, -1);
while (open_list_count > 0) {
i = get_min_f_node();
if (i == -1) {
return -1;
}
current_node = open_list[i];
if (current_node.x == end_x && current_node.y == end_y) {
return 1;
}
open_list[i] = open_list[open_list_count - 1];
open_list_count--;
close_list[close_list_count] = current_node;
close_list_count++;
for (new_x = current_node.x - 1; new_x <= current_node.x + 1; new_x++) {
for (new_y = current_node.y - 1; new_y <= current_node.y + 1; new_y++) {
if (new_x < 0 || new_x >= MAP_WIDTH || new_y < 0 || new_y >= MAP_HEIGHT) {
continue;
}
if (new_x == current_node.x && new_y == current_node.y) {
continue;
}
if (map[new_y][new_x] == 1) {
continue;
}
if (in_close_list(new_x, new_y)) {
continue;
}
g = current_node.g + distance(current_node.x, current_node.y, new_x, new_y);
h = distance(new_x, new_y, end_x, end_y);
f = g + h;
if (!in_open_list(new_x, new_y)) {
add_to_open_list(new_x, new_y, g, h, current_node.x, current_node.y);
}
}
}
}
return -1;
}
int main() {
int start_x = 1;
int start_y = 1;
int end_x = 8;
int end_y = 8;
if (find_path(start_x, start_y, end_x, end_y) == 1) {
printf("path found!\n");
} else {
printf("path not found!\n");
}
return 0;
}
```
以上代码仅供参考,具体实现需要根据实际需求进行修改和完善。
阅读全文
相关推荐
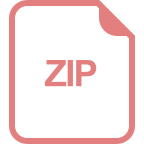



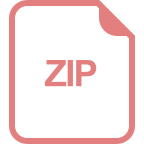
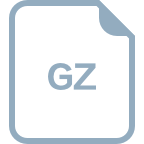
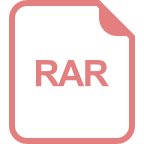
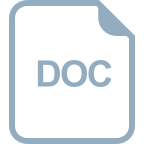
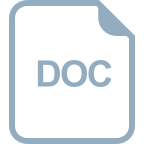
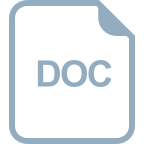
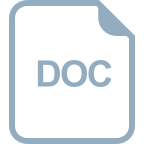
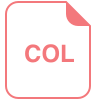
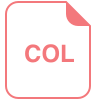



