设计以下问题算法:3. Single-source shortest paths. The following is the adjacency matrix, vertex A is the source. A B C D E A -1 3 B 3 2 2 C D 1 5 E -3
时间: 2024-02-10 19:19:58 浏览: 134
这是一个经典的单源最短路径问题,可以使用 Dijkstra 算法或者 Bellman-Ford 算法进行求解。下面给出两种算法的伪代码:
Dijkstra 算法:
1. 初始化距离数组 dist,将源点 A 的距离设为 0,其他点的距离设为无穷大。
2. 创建一个空的集合 visited,用于存放已经确定最短路径的点。
3. while visited 集合不包含所有点:
4. 在未访问的点中找到距离源点最近的点 u,将 u 加入 visited 集合。
5. 对于 u 的每一个邻居 v,如果从源点 A 到 v 的距离比从源点 A 到 u 再到 v 的距离更短,则更新 dist[v] 的值为更小的距离。
6. 返回 dist 数组,其中 dist[i] 表示从源点 A 到点 i 的最短路径长度。
Bellman-Ford 算法:
1. 初始化距离数组 dist,将源点 A 的距离设为 0,其他点的距离设为无穷大。
2. 重复执行以下操作 V-1 次(V 是点的个数):
3. 对于每一条边 (u,v),如果从源点 A 到 u 的距离加上边权 w(u,v) 小于 dist[v],则更新 dist[v] 的值为更小的距离。
4. 检查是否存在从源点 A 出发的负权回路。如果存在,则无法求出最短路径。
5. 返回 dist 数组,其中 dist[i] 表示从源点 A 到点 i 的最短路径长度。
相关问题
The function Dijkstra is to find the shortest path from Vertex S to every other vertices in a given Graph. The distances are stored in dist[], and path[] records the paths. The MGraph is defined as the following: typedef struct GNode *PtrToGNode; struct GNode{ int Nv; /* Number of vertices */ int Ne; /* Number of edges */ WeightType G[MaxVertexNum][MaxVertexNum]; /* adjacency matrix */ }; typedef PtrToGNode MGraph; void Dijkstra( MGraph Graph, int dist[], int path[], Vertex S ) { int collected[MaxVertexNum]; Vertex V, W; for ( V=0; V<Graph->Nv; V++ ) { dist[V] = Graph->G[S][V]; path[V] = -1; collected[V] = false; } dist[S] = 0; collected[S] = true; while (1) { V = FindMinDist( Graph, dist, collected ); if ( V==ERROR ) break; collected[V] = true; for( W=0; W<Graph->Nv; W++ ) if ( collected[W]==false && Graph->G[V][W]<INFINITY ) { if ( ) { dist[W] = ; path[W] = ; } } } /* end while */ }
在Dijkstra函数中,缺少一个条件判断语句,用来判断当前节点W是否可以通过V到达S的距离更短。应该在if语句中加上这个条件判断,如下所示:
if ( collected[W]==false && Graph->G[V][W]<INFINITY ) {
if ( dist[V] + Graph->G[V][W] < dist[W] ) {
dist[W] = dist[V] + Graph->G[V][W];
path[W] = V;
}
}
这样就可以在更新dist[W]和path[W]的时候,同时判断是否需要更新最短路径。
matlab代码利用Dijkstra算法求有向图最短路代码
下面是一个简单的 Matlab 程序,用 Dijkstra 算法求解有向图的最短路径问题:
```matlab
function [dist, path] = Dijkstra(A, s)
% A - adjacency matrix of the directed graph
% s - the starting vertex
% dist - distances from the starting vertex to all other vertices
% path - shortest paths from the starting vertex to all other vertices
n = size(A, 1);
dist = inf(1, n);
dist(s) = 0;
path = cell(1, n);
for i = 1:n
path{i} = [s];
end
for i = 1:n-1
[~, u] = min(dist);
dist(u) = inf;
for v = 1:n
if A(u, v) > 0
alt = dist(u) + A(u, v);
if alt < dist(v)
dist(v) = alt;
path{v} = [path{u}, v];
end
end
end
end
```
其中,`A` 是一个邻接矩阵,表示有向图的连接情况,`s` 是起始节点的编号。程序返回两个变量:`dist` 表示起始节点到其他节点的最短距离,`path` 表示起始节点到其他节点的最短路径。
阅读全文
相关推荐
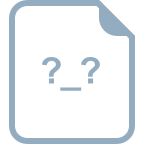
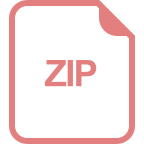
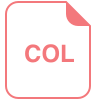
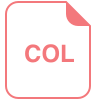
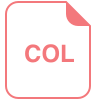
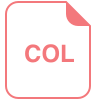
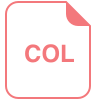
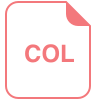
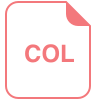
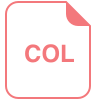
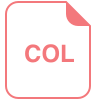
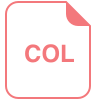
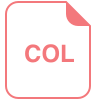
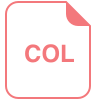
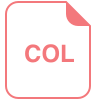