openssl使用gcm模式解密,c实现
时间: 2024-05-02 13:23:16 浏览: 11
以下是C语言中使用openssl库解密GCM模式的代码示例:
```
#include <stdio.h>
#include <openssl/evp.h>
#include <openssl/aes.h>
// 填充密文,密钥和IV
const unsigned char ciphertext[] = { 0x64, 0x5b, 0xa6, 0xe8, 0x45, 0xb, 0xf5, 0x7a, 0x77, 0xd1, 0xea, 0xf3, 0x83, 0xb3, 0x2a, 0x14, 0xdb, 0x2f, 0x7, 0xaf, 0xce, 0xba, 0x4, 0x88, 0xd4, 0xe4, 0xda, 0x31, 0xc9, 0xf4, 0x4, 0xcc };
const unsigned char key[] = { 0x10, 0x30, 0x50, 0x70, 0x90, 0xb0, 0xd0, 0xf0, 0x1, 0x3, 0x5, 0x7, 0x9, 0xb, 0xd, 0xf };
const unsigned char iv[] = { 0x1, 0x2, 0x3, 0x4, 0x5, 0x6, 0x7, 0x8, 0x9, 0xa, 0xb, 0xc, 0xd, 0xe, 0xf, 0x10 };
int main(void) {
EVP_CIPHER_CTX *ctx;
int len, plaintext_len;
unsigned char plaintext[32];
// 创建并初始化EVP_CIPHER_CTX结构体
if(!(ctx = EVP_CIPHER_CTX_new())) {
printf("Error: EVP_CIPHER_CTX_new failed!\n");
return 1;
}
// 设置解密算法为AES-256-GCM,设置密钥和IV,并初始化EVP_CIPHER_CTX结构体
if(1 != EVP_DecryptInit_ex(ctx, EVP_aes_256_gcm(), NULL, NULL, NULL)) {
printf("Error: EVP_DecryptInit_ex failed!\n");
return 1;
}
if(1 != EVP_DecryptInit_ex(ctx, NULL, NULL, key, iv)) {
printf("Error: EVP_DecryptInit_ex failed!\n");
return 1;
}
// 填充密文,解密并填充明文
if(1 != EVP_DecryptUpdate(ctx, plaintext, &len, ciphertext, sizeof(ciphertext))) {
printf("Error: EVP_DecryptUpdate failed!\n");
return 1;
}
plaintext_len = len;
// 最后一次解密明文
if(1 != EVP_DecryptFinal_ex(ctx, plaintext + len, &len)) {
printf("Error: EVP_DecryptFinal_ex failed!\n");
return 1;
}
plaintext_len += len;
// 输出解密后的明文
printf("Decrypted text is:\n");
for(int i = 0; i < plaintext_len; i++) {
printf("%02x ", plaintext[i]);
}
printf("\n");
// 释放EVP_CIPHER_CTX结构体
EVP_CIPHER_CTX_free(ctx);
return 0;
}
```
使用以上代码进行编译并运行即可解密GCM加密的密文。在代码中,我们通过openssl库中的EVP接口实现了AES-256-GCM解密。首先创建并初始化EVP_CIPHER_CTX结构体,然后设置解密算法为AES-256-GCM,设置密钥和IV,并初始化EVP_CIPHER_CTX结构体。最后,调用EVP_DecryptUpdate函数和EVP_DecryptFinal_ex函数即可完成解密过程。
相关推荐
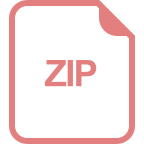
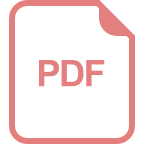
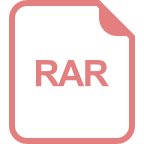














