flink自定义数据落地到hive
时间: 2023-05-25 09:05:23 浏览: 401
要在Flink中自定义数据落地到Hive,需要进行以下步骤:
1. 编写自定义Hive表工厂
使用自定义Hive表工厂,您可以将表定义转换为Hive表。您可以通过实现`TableFactory`和`TableSinkFactory`接口来编写自定义Hive表工厂。
2. 实现TableSink接口
TableSink接口提供了将表的内容插入到输出中(如Hive表)的方法。为了自定义数据落地到Hive,您需要创建一个实现TableSink接口的类,并实现TableSink的方法。例如,您可以使用`HiveTableSink`类作为模板,然后自己实现。
3. 配置HiveTableSink
配置HiveTableSink时,您需要指定Hive表的名称、列名和类型。您还需要指定Hive的连接参数,以便将数据插入到Hive表中。
4. 使用自定义HiveTableSink插入数据
在Flink代码中,您需要使用自定义HiveTableSink插入数据。您可以使用Flink API(例如insertInto方法)来将数据插入到Hive表中。
总结
在自定义数据落地到Hive时,您需要编写自定义Hive表工厂、实现TableSink接口、配置HiveTableSink和使用自定义HiveTableSink来插入数据。这需要一些Flink和Hive的知识,但一旦您理解它,就可以自定义数据落地到Hive。
相关问题
flink自定义数据落地到hive代码示例
以下是一个简单的 Flink 代码示例,它将自定义数据流落地到 Hive 表中:
```java
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.api.common.serialization.SimpleStringEncoder;
import org.apache.flink.api.common.serialization.SimpleStringSchema;
import org.apache.flink.core.fs.Path;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.functions.sink.filesystem.StreamingFileSink;
import org.apache.flink.streaming.connectors.kafka.FlinkKafkaConsumer;
import org.apache.flink.table.api.EnvironmentSettings;
import org.apache.flink.table.api.Table;
import org.apache.flink.table.api.bridge.java.StreamTableEnvironment;
import org.apache.flink.table.catalog.hive.HiveCatalog;
import org.apache.hadoop.hive.conf.HiveConf;
import java.util.Properties;
public class FlinkHiveSink {
public static void main(String[] args) throws Exception {
// 设置执行环境
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
env.setParallelism(1);
// 设置 Table 环境
EnvironmentSettings settings = EnvironmentSettings.newInstance().useBlinkPlanner().inStreamingMode().build();
StreamTableEnvironment tableEnv = StreamTableEnvironment.create(env, settings);
// 设置 Hive catalog
String catalogName = "my_hive_catalog";
String defaultDatabase = "default";
String hiveConfDir = "/etc/hadoop/conf";
HiveConf hiveConf = new HiveConf();
hiveConf.addResource(new Path(hiveConfDir + "/hive-site.xml"));
HiveCatalog hiveCatalog = new HiveCatalog(catalogName, defaultDatabase, hiveConf);
tableEnv.registerCatalog(catalogName, hiveCatalog);
// 设置 Kafka 数据源
Properties kafkaProps = new Properties();
kafkaProps.setProperty("bootstrap.servers", "<your-bootstrap-servers>");
kafkaProps.setProperty("group.id", "<your-group-id>");
FlinkKafkaConsumer<String> kafkaConsumer = new FlinkKafkaConsumer<>("my-topic", new SimpleStringSchema(), kafkaProps);
DataStream<String> dataStream = env.addSource(kafkaConsumer);
// 将数据流转换为 Table
Table table = tableEnv.fromDataStream(dataStream, "value");
// 定义输出的 Table
String tableName = "my_hive_table";
String createTableStmt = String.format("CREATE TABLE IF NOT EXISTS %s (value STRING) STORED AS TEXTFILE", tableName);
tableEnv.executeSql(createTableStmt);
tableEnv.useCatalog(catalogName);
tableEnv.useDatabase(defaultDatabase);
tableEnv.createTemporaryView("temp_table", table);
String insertStmt = String.format("INSERT INTO %s SELECT * FROM temp_table", tableName);
tableEnv.executeSql(insertStmt);
// 将数据流落地到 HDFS
StreamingFileSink<String> sink = StreamingFileSink
.forRowFormat(new Path("hdfs://<your-hdfs-path>"), new SimpleStringEncoder<String>("UTF-8"))
.build();
dataStream.map(new MapFunction<String, String>() {
@Override
public String map(String value) throws Exception {
return value;
}
}).addSink(sink);
// 执行任务
env.execute("Flink Hive Sink Example");
}
}
```
在这个示例中,我们首先设置了执行环境和 Table 环境。然后,我们设置了 Hive catalog 和 Kafka 数据源。接着,我们将数据流转换为 Table 并定义要输出的 Table。最后,我们使用 Flink 的 StreamingFileSink 将数据写入 HDFS 中。
flink 自定义批量落地hivesink
要将 Flink 中的数据批量写入到 Hive 中,可以使用 Flink 的 `StreamingFileSink` 将数据先写入到本地文件系统中,然后再使用 Flink 的 `HadoopOutputFormat` 将数据批量写入到 Hive 中。
以下是一个示例代码:
```
// 创建 StreamingFileSink
StreamingFileSink<String> sink = StreamingFileSink
.forRowFormat(new Path("/path/to/local/files"), new SimpleStringEncoder<String>("UTF-8"))
.withRollingPolicy(
DefaultRollingPolicy.builder()
.withRolloverInterval(TimeUnit.MINUTES.toMillis(15))
.withInactivityInterval(TimeUnit.MINUTES.toMillis(5))
.withMaxPartSize(1024 * 1024 * 1024)
.build())
.build();
// 将数据写入到 StreamingFileSink 中
DataStream<String> dataStream = ...
dataStream.addSink(sink);
// 创建 HadoopOutputFormat
HiveConf conf = new HiveConf();
HadoopOutputFormat<Text, NullWritable> outputFormat = new HiveOutputFormat<Text, NullWritable>(conf, "myTable");
// 使用 HadoopOutputFormat 将数据批量写入到 Hive 中
Job job = Job.getInstance();
HadoopOutputFormat.setOutput(job, outputFormat);
FileInputFormat.setInputPaths(job, "/path/to/local/files");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_TABLE, "myTable");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_SCHEMA, "col1 STRING, col2 INT");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.job.reduces", "1");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.job.name", "myJob");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.output.fileoutputformat.outputdir", "/tmp/hive-output");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.output.fileoutputformat.compress", "false");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.output.fileoutputformat.compress.type", "NONE");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.output.fileoutputformat.compress.codec", "org.apache.hadoop.io.compress.DefaultCodec");
outputFormat.getConfiguration().set(TableOutputFormat.OUTPUT_CONF_PREFIX + "mapreduce.output.fileoutputformat.compress.codec.null", "org.apache.hadoop.io.compress.DefaultCodec");
job.waitForCompletion(true);
```
首先,创建一个 `StreamingFileSink`,将数据写入到本地文件系统中。然后,创建一个 `HadoopOutputFormat`,将数据批量写入到 Hive 中。需要注意的是,需要在 `HadoopOutputFormat` 中指定要写入的表名、表结构和输出路径等参数。在示例代码中,输出路径为 `/tmp/hive-output`,可以根据实际情况进行修改。
阅读全文
相关推荐
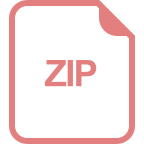
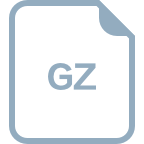













