使用Java实现dijkstra最短路径算法
时间: 2023-10-16 16:11:46 浏览: 93
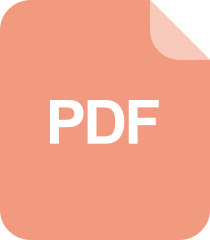
java实现dijkstra最短路径寻路算法

Dijkstra算法是一种单源最短路径算法,它可以求出一个节点到其他所有节点的最短路径。下面是使用Java实现Dijkstra算法的示例代码:
```java
import java.util.*;
public class DijkstraAlgorithm {
private static final int NO_PARENT = -1;
public static void dijkstra(int[][] adjacencyMatrix, int startVertex) {
int nVertices = adjacencyMatrix[0].length;
int[] shortestDistances = new int[nVertices];
boolean[] visited = new boolean[nVertices];
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
shortestDistances[vertexIndex] = Integer.MAX_VALUE;
visited[vertexIndex] = false;
}
shortestDistances[startVertex] = 0;
int[] parents = new int[nVertices];
parents[startVertex] = NO_PARENT;
for (int i = 1; i < nVertices; i++) {
int nearestVertex = -1;
int shortestDistance = Integer.MAX_VALUE;
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
if (!visited[vertexIndex] && shortestDistances[vertexIndex] < shortestDistance) {
nearestVertex = vertexIndex;
shortestDistance = shortestDistances[vertexIndex];
}
}
visited[nearestVertex] = true;
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
int edgeDistance = adjacencyMatrix[nearestVertex][vertexIndex];
if (edgeDistance > 0 && ((shortestDistance + edgeDistance) < shortestDistances[vertexIndex])) {
parents[vertexIndex] = nearestVertex;
shortestDistances[vertexIndex] = shortestDistance + edgeDistance;
}
}
}
printSolution(startVertex, shortestDistances, parents);
}
private static void printSolution(int startVertex, int[] distances, int[] parents) {
int nVertices = distances.length;
System.out.print("Vertex\t Distance\tPath");
for (int vertexIndex = 0; vertexIndex < nVertices; vertexIndex++) {
if (vertexIndex != startVertex) {
System.out.print("\n" + startVertex + " -> ");
System.out.print(vertexIndex + " \t\t ");
System.out.print(distances[vertexIndex] + "\t\t");
printPath(vertexIndex, parents);
}
}
}
private static void printPath(int currentVertex, int[] parents) {
if (currentVertex == NO_PARENT) {
return;
}
printPath(parents[currentVertex], parents);
System.out.print(currentVertex + " ");
}
public static void main(String[] args) {
int[][] adjacencyMatrix = { { 0, 4, 0, 0, 0, 0, 0, 8, 0 },
{ 4, 0, 8, 0, 0, 0, 0, 11, 0 },
{ 0, 8, 0, 7, 0, 4, 0, 0, 2 },
{ 0, 0, 7, 0, 9, 14, 0, 0, 0 },
{ 0, 0, 0, 9, 0, 10, 0, 0, 0 },
{ 0, 0, 4, 14, 10, 0, 2, 0, 0 },
{ 0, 0, 0, 0, 0, 2, 0, 1, 6 },
{ 8, 11, 0, 0, 0, 0, 1, 0, 7 },
{ 0, 0, 2, 0, 0, 0, 6, 7, 0 } };
dijkstra(adjacencyMatrix, 0);
}
}
```
上述代码中,使用邻接矩阵来表示图,其中0表示两个节点之间没有边,正整数表示两个节点之间有边,边的权重就是这个正整数。在执行dijkstra方法之前,需要指定起始节点的索引。最终输出的结果包括每个节点到起始节点的最短路径长度以及路径本身。
阅读全文
相关推荐
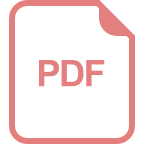
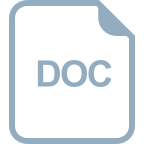
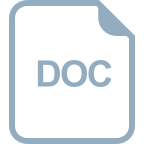
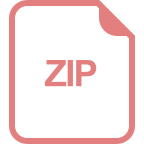
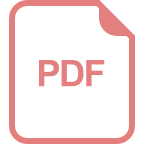
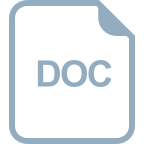

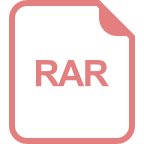
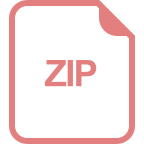
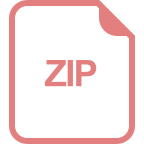
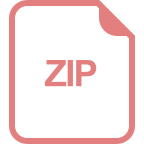
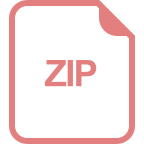
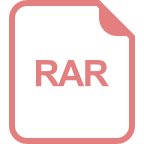
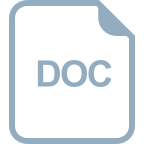
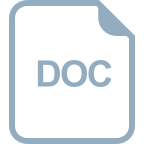