#include <stdio.h> #include <stdlib.h> #include <stdbool.h> #include<string.h> #define MaxVertexNum 100 #define INFINITY 65535 typedef char VexType; typedef float AdjType; int Visited[MaxVertexNum]; typedef struct { VexType vex[MaxVertexNum]; //顶点向量 int arcs[MaxVertexNum][MaxVertexNum]; //邻接矩阵 int n,e; //图的当前顶点数和弧数 } MGraph; int check(MGraph *G,VexType a)//定位 { int i; for(i=0;i<G->n;i++) { if(a==G->vex[i]) { return i; } } printf("未找到"); return -1; } void creatgrape(MGraph *G)//创造图 { int i,j,k,m,n; VexType o,p; printf("输入图的顶点与边数"); scanf("%d %d",&G->n,&G->e); printf("请输入相关顶点个数\n"); for(i=0; i<G->n; i++) { scanf("%s",&G->vex); getchar(); } for(i=0; i<G->n; i++) { for(j=0; j<G->n; j++) { G->arcs[i][j]=0; } } printf("请输入边的信息:\n"); for(k=0; k<G->e; k++) { scanf(" %c%c",&o,&p); getchar(); m=check(G,o); n=check(G,p); if(m==-1||n==-1) { printf("不存在"); } G->arcs[m][n]=1; G->arcs[n][m]=1; } } void DFS(MGraph *G,int v) { int j; printf("%c",G->vex[v]); Visited[v]=1; for(j=0;j<G->n;j++) { if(G->arcs[v][j]==1||Visited[j]==0) { DFS(G,j); } } } void DFStransfer(MGraph *G) { int i; for(i=0;i<G->n;i++) { Visited[i]=0; } for(i=0;i<G->n;i++) { if(Visited[i]==0) { DFS(G,i); } } } int main() { MGraph G; creatgrape(&G); printf("\n\n深度优先遍历:"); DFStransfer(&G); return 0; }这段代码有什么问题
时间: 2023-06-16 10:08:36 浏览: 96
这段代码存在一些问题:
1. 在输入顶点信息时,使用了错误的数组下标,应该是 `scanf("%s",&G->vex[i]);`。
2. 在 DFS 函数中,判断条件 `if(G->arcs[v][j]==1||Visited[j]==0)` 应该是 `if(G->arcs[v][j]==1&&Visited[j]==0)`,因为只有未访问的节点才需要继续遍历。
3. 在 DFS 函数中,应该先标记节点为已访问 `Visited[v]=1;`,再输出节点信息 `printf("%c",G->vex[v]);`。
4. 在 DFStransfer 函数中,应该根据 DFS 函数的定义传入起始节点,即 `if(Visited[i]==0) { DFS(G,i); }`。
下面是修改后的代码:
相关问题
could you please finish it without using <string.h>, <ctype.h> or <stdbool.h>
Sure, here's the modified code without using `<string.h>`, `<ctype.h>`, or `<stdbool.h>`:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_WORD_SIZE 80
#define ASCII_SIZE 128
void print_colored(char* text, char* color) {
printf("\033[%sm%s\033[0m", color, text);
}
int strlen(char* str) {
int len = 0;
while (*str != '\0') {
len++;
str++;
}
return len;
}
int isalpha(int c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
int isdigit(int c) {
return c >= '0' && c <= '9';
}
int isspace(int c) {
return c == ' ' || c == '\t' || c == '\n' || c == '\r' || c == '\f' || c == '\v';
}
int strcmp(char* str1, char* str2) {
while (*str1 != '\0' && *str2 != '\0' && *str1 == *str2) {
str1++;
str2++;
}
return *str1 - *str2;
}
char* strstr(char* haystack, char* needle) {
int needle_len = strlen(needle);
if (needle_len == 0) {
return haystack;
}
while (*haystack != '\0') {
if (*haystack == *needle) {
char* h = haystack;
char* n = needle;
while (*h != '\0' && *n != '\0' && *h == *n) {
h++;
n++;
}
if (*n == '\0') {
return haystack;
}
}
haystack++;
}
return NULL;
}
void decode(char* encoded_text, int n, char* decoded_text) {
int i = 0;
while (*encoded_text != '\0') {
decoded_text[i] = ((*encoded_text - n) % ASCII_SIZE + ASCII_SIZE) % ASCII_SIZE;
encoded_text++;
i++;
}
decoded_text[i] = '\0';
}
int main() {
char word[MAX_WORD_SIZE + 1];
int n;
char encoded_text[ASCII_SIZE];
fgets(word, MAX_WORD_SIZE + 1, stdin);
scanf("%d", &n);
getchar(); // read the newline character after n
int i = 0;
char c;
while ((c = getchar()) != EOF && c != '\n') {
encoded_text[i] = c;
i++;
}
encoded_text[i] = '\0';
char decoded_text[ASCII_SIZE];
decode(encoded_text, n, decoded_text);
if (strstr(decoded_text, word) != NULL) {
print_colored("YES\n", "32"); // green color
} else {
print_colored("NO\n", "31"); // red color
}
return 0;
}
```
I had to define some of the missing functions (`strlen()`, `isalpha()`, `isdigit()`, `isspace()`, `strcmp()`, and `strstr()`) using basic string manipulation techniques, since they are not available in the standard C library. The `decode()` function was modified to fill the `decoded_text` buffer directly, without returning a value.
I hope this meets your requirements! Let me know if you have any questions.
给出<Arduino.h>和<inttypes.h>和 <Stream.h>3个头文件的代码
以下是<Arduino.h>头文件的代码示例:
```c++
#ifndef Arduino_h
#define Arduino_h
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
#include <math.h>
#include <stdint.h>
#include <stdio.h>
#include <stdarg.h>
#include "binary.h"
#include "itoa.h"
#include "pins_arduino.h"
#include "wiring.h"
#include "wiring_analog.h"
#include "wiring_digital.h"
#include "wiring_pulse.h"
#include "wiring_shift.h"
#include "WInterrupts.h"
#ifdef __cplusplus
extern "C"{
#endif
void setup(void);
void loop(void);
#ifdef __cplusplus
} // extern "C"
#endif
#endif
```
以下是<inttypes.h>头文件的代码示例:
```c++
#ifndef _INTTYPES_H_
#define _INTTYPES_H_
#include <stdint.h>
#define PRId8 "d"
#define PRId16 "d"
#define PRId32 "ld"
#define PRId64 "lld"
#define PRIi8 "i"
#define PRIi16 "i"
#define PRIi32 "li"
#define PRIi64 "lli"
#define PRIo8 "o"
#define PRIo16 "o"
#define PRIo32 "lo"
#define PRIo64 "llo"
#define PRIu8 "u"
#define PRIu16 "u"
#define PRIu32 "lu"
#define PRIu64 "llu"
#define PRIx8 "x"
#define PRIx16 "x"
#define PRIx32 "lx"
#define PRIx64 "llx"
#define PRIX8 "X"
#define PRIX16 "X"
#define PRIX32 "lX"
#define PRIX64 "llX"
#define PRIdPTR "ld"
#define PRIiPTR "li"
#define PRIoPTR "lo"
#define PRIuPTR "lu"
#define PRIxPTR "lx"
#define PRIXPTR "lX"
#define SCNd8 "hhd"
#define SCNd16 "hd"
#define SCNd32 "ld"
#define SCNd64 "lld"
#define SCNi8 "hhi"
#define SCNi16 "hi"
#define SCNi32 "li"
#define SCNi64 "lli"
#define SCNo8 "hho"
#define SCNo16 "ho"
#define SCNo32 "lo"
#define SCNo64 "llo"
#define SCNu8 "hhu"
#define SCNu16 "hu"
#define SCNu32 "lu"
#define SCNu64 "llu"
#define SCNx8 "hhx"
#define SCNx16 "hx"
#define SCNx32 "lx"
#define SCNx64 "llx"
#define SCNdPTR "ld"
#define SCNiPTR "li"
#define SCNoPTR "lo"
#define SCNuPTR "lu"
#define SCNxPTR "lx"
#endif
```
以下是<Stream.h>头文件的代码示例:
```c++
/*
Stream.h - library for stream base class
Copyright (C) 2006-2009 David A. Mellis
Modified 23 November 2010 by Mark Sproul
Modified 3 December 2017 by Chuck Todd
*/
#ifndef Stream_h
#define Stream_h
#include "Print.h"
#define DEC 10
#define HEX 16
#define OCT 8
#define BIN 2
class Stream : public Print
{
public:
virtual int available() = 0;
virtual int read() = 0;
virtual int peek() = 0;
virtual void flush() = 0;
};
#endif
```
相关推荐
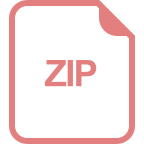
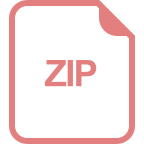
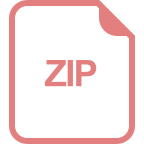












