写一个aes加密算法的代码
时间: 2023-04-03 09:03:25 浏览: 118
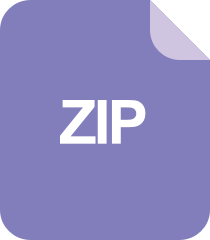
密码学之AES加密算法代码
以下是一个简单的 AES 加密算法的代码示例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#define Nb 4
#define Nk 4
#define Nr 10
typedef uint8_t state_t[4][4];
static const uint8_t sbox[256] = {
// S-box
};
static const uint8_t rsbox[256] = {
// Inverse S-box
};
static const uint8_t Rcon[11] = {
// Round constants
};
static void sub_bytes(state_t *state)
{
// SubBytes step
}
static void shift_rows(state_t *state)
{
// ShiftRows step
}
static void mix_columns(state_t *state)
{
// MixColumns step
}
static void add_round_key(uint8_t round, state_t *state, const uint8_t *key)
{
// AddRoundKey step
}
static void key_expansion(const uint8_t *key, uint8_t *w)
{
// KeyExpansion routine
}
void aes_encrypt(const uint8_t *in, uint8_t *out, const uint8_t *key)
{
state_t state;
uint8_t w[4 * Nb * (Nr + 1)];
key_expansion(key, w);
memcpy(state, in, 4 * Nb);
add_round_key(0, &state, key);
for (uint8_t round = 1; round < Nr; ++round) {
sub_bytes(&state);
shift_rows(&state);
mix_columns(&state);
add_round_key(round, &state, w + round * 4 * Nb);
}
sub_bytes(&state);
shift_rows(&state);
add_round_key(Nr, &state, w + Nr * 4 * Nb);
memcpy(out, state, 4 * Nb);
}
int main(void)
{
uint8_t in[16] = { /* Input data */ };
uint8_t out[16];
uint8_t key[16] = { /* Key */ };
aes_encrypt(in, out, key);
for (int i = 0; i < 16; ++i) {
printf("%02x ", out[i]);
}
printf("\n");
return 0;
}
阅读全文
相关推荐
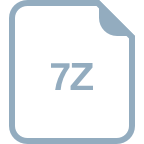
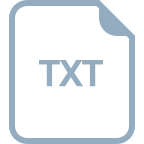















