opencv threshold
时间: 2023-09-19 12:06:44 浏览: 76
### 回答1:
In OpenCV, thresholding is a common image processing technique used to separate image regions based on their pixel intensity values. The basic idea is to convert a grayscale image into a binary image, where each pixel is either black or white, based on a specified threshold value.
OpenCV provides a function called `cv2.threshold` for thresholding an image. Here is the basic syntax of the `cv2.threshold` function:
```python
ret, thresh = cv2.threshold(src, thresh, maxval, type)
```
where:
- `src`: Input image (grayscale).
- `thresh`: Threshold value, which is used to classify the pixel values as black or white.
- `maxval`: Maximum value that is assigned to a pixel if its value is greater than the threshold value. This is usually set to 255.
- `type`: Type of thresholding to be applied. This can be one of the following:
- `cv2.THRESH_BINARY`: Pixels with intensity value greater than the threshold value are set to `maxval`, and all other pixels are set to 0.
- `cv2.THRESH_BINARY_INV`: Inverted binary thresholding, where pixels with intensity value greater than the threshold value are set to 0, and all other pixels are set to `maxval`.
- `cv2.THRESH_TRUNC`: Pixels with intensity value greater than the threshold value are set to the threshold value, and all other pixels remain unchanged.
- `cv2.THRESH_TOZERO`: Pixels with intensity value less than the threshold value are set to 0, and all other pixels remain unchanged.
- `cv2.THRESH_TOZERO_INV`: Inverted thresholding, where pixels with intensity value less than the threshold value are set to `maxval`, and all other pixels are set to 0.
The `cv2.threshold` function returns two values:
- `ret`: The threshold value used.
- `thresh`: The thresholded image.
Here's an example of how to use the `cv2.threshold` function to perform binary thresholding on an input image:
```python
import cv2
# Load input image (grayscale)
img = cv2.imread('input.jpg', 0)
# Apply binary thresholding
thresh_value = 128
max_value = 255
ret, thresh = cv2.threshold(img, thresh_value, max_value, cv2.THRESH_BINARY)
# Display thresholded image
cv2.imshow('Thresholded Image', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
In this example, we load a grayscale image and apply binary thresholding with a threshold value of 128. The resulting thresholded image is displayed using the `cv2.imshow` function.
### 回答2:
OpenCV中的threshold函数是图像处理中常用的阈值化方法。阈值化是一种将图像分割成两种或多种不同亮度或颜色的区域的方法。
阈值化的原理是将图像中的像素值与一个预设的阈值进行比较,如果像素值大于阈值,则将像素值设置为一个给定的最大值(一般为255),如果像素值小于阈值,则将像素值设置为一个给定的最小值(一般为0)。通过这种方式,可以将图像中的像素按照亮度或颜色进行分割。
在OpenCV中,threshold函数的使用方法如下:
cv2.threshold(src, thresh, maxval, type[, dst])
参数说明:
- src:待处理的输入图像。
- thresh:设定的阈值。
- maxval:像素值大于阈值时设置的最大值。
- type:阈值化的类型,包括:
- cv2.THRESH_BINARY:二值化阈值化,大于阈值的像素设置为maxval,小于阈值的像素值设置为0。
- cv2.THRESH_BINARY_INV:反二值化阈值化,大于阈值的像素值设为0,小于阈值的像素值设为maxval。
- cv2.THRESH_TRUNC:截断阈值化,大于阈值的像素值设置为阈值,小于阈值的像素值保持不变。
- cv2.THRESH_TOZERO:阈值化为0,大于阈值的像素值保持不变,小于阈值的像素值设置为0。
- cv2.THRESH_TOZERO_INV:反阈值化为0,大于阈值的像素值设置为0,小于阈值的像素值保持不变。
- dst:输出图像。
通过调整阈值和阈值化的类型,可以得到不同的效果。阈值化可以应用于多种图像处理任务,比如目标检测、图像分割、边缘提取等。
### 回答3:
OpenCV是一个开源的计算机视觉库。其中的threshold函数是实现图像二值化的一个重要工具。
二值化是指将图像转换成只有两种值的图像,一般为黑白图像或者灰度图像。而threshold函数就是实现图像二值化的函数之一。它的基本原理是将图像的每个像素点与给定的阈值进行比较,如果大于阈值则设为最大值,如果小于等于阈值则设为最小值,从而实现二值化。
threshold函数的具体调用方式为:
cv2.threshold(src, thresh, maxval, type[, dst]) -> retval, threshold
src代表输入图像,一般为灰度图像;
thresh为阈值;
maxval为图像中像素点的最大值;
type为二值化类型,有以下几种类型可选:
cv2.THRESH_BINARY:大于阈值的像素点设为maxval,小于等于阈值的像素点设为0;
cv2.THRESH_BINARY_INV:大于阈值的像素点设为0,小于等于阈值的像素点设为maxval;
cv2.THRESH_TRUNC:大于阈值的像素点设为阈值,小于等于阈值的像素点保持不变;
cv2.THRESH_TOZERO:大于阈值的像素点保持不变,小于等于阈值的像素点设为0;
cv2.THRESH_TOZERO_INV:大于阈值的像素点设为0,小于等于阈值的像素点保持不变;
retval为阈值,threshold是一个输出参数,表示二值化后的图像。
通过使用threshold函数,可以将图像进行二值化处理,提取出感兴趣的目标区域,同时也可以去除图像中的噪声,方便进行后续的图像分析和处理。
相关推荐
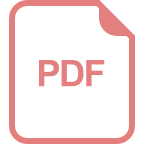















