编写一段Python代码实现NSGA2
时间: 2023-06-08 22:08:14 浏览: 129
好的,以下是一段实现NSGA2算法的Python代码:
```python
import copy
import random
class NSGA2:
def __init__(self, fitness_function, num_generations, population_size, crossover_prob, mutation_prob):
self.fitness_function = fitness_function
self.num_generations = num_generations
self.population_size = population_size
self.crossover_prob = crossover_prob
self.mutation_prob = mutation_prob
def run(self):
population = self.initialize_population(self.population_size)
for generation in range(self.num_generations):
fronts = self.fast_non_dominated_sort(population)
new_population = []
front_index = 0
while len(new_population) + len(fronts[front_index]) <= self.population_size:
for individual in fronts[front_index]:
new_population.append(individual)
front_index += 1
if len(new_population) == self.population_size - 1:
new_population.append(random.choice(fronts[front_index]))
while len(new_population) < self.population_size:
parent_1 = self.tournament_selection(population)
parent_2 = self.tournament_selection(population)
child_1, child_2 = self.crossover(parent_1, parent_2)
if random.random() < self.mutation_prob:
child_1 = self.mutate(child_1)
if random.random() < self.mutation_prob:
child_2 = self.mutate(child_2)
new_population.append(child_1)
if len(new_population) < self.population_size:
new_population.append(child_2)
population = new_population
return population
def initialize_population(self, population_size):
population = []
for _ in range(population_size):
individual = []
for _ in range(2):
gene = random.uniform(0.0, 1.0)
individual.append(gene)
population.append(individual)
return population
def fast_non_dominated_sort(self, population):
fronts = [[]]
individual_rank = {}
individual_dominated_set = {}
for individual in population:
individual_dominated_set[individual] = set()
individual_rank[individual] = 0
for other_individual in population:
if self.is_dominant(individual, other_individual):
individual_dominated_set[individual].add(other_individual)
elif self.is_dominant(other_individual, individual):
individual_rank[individual] += 1
if individual_rank[individual] == 0:
fronts[0].append(individual)
current_front_index = 0
while len(fronts[current_front_index]) > 0:
next_front = []
for individual in fronts[current_front_index]:
for other_individual in individual_dominated_set[individual]:
individual_rank[other_individual] -= 1
if individual_rank[other_individual] == 0:
next_front.append(other_individual)
current_front_index += 1
fronts.append(next_front)
return fronts
def is_dominant(self, individual_1, individual_2):
fitness_1 = self.fitness_function(individual_1)
fitness_2 = self.fitness_function(individual_2)
is_better = False
for i in range(len(fitness_1)):
if fitness_1[i] > fitness_2[i]:
return False
elif fitness_1[i] < fitness_2[i]:
is_better = True
return is_better
def tournament_selection(self, population):
tournament_size = int(len(population) / 10)
tournament = random.sample(population, tournament_size)
best_individual = None
best_fitness = None
for individual in tournament:
fitness = self.fitness_function(individual)
if best_individual is None or fitness < best_fitness:
best_individual = individual
best_fitness = fitness
return best_individual
def crossover(self, parent_1, parent_2):
child_1 = copy.deepcopy(parent_1)
child_2 = copy.deepcopy(parent_2)
if random.random() < self.crossover_prob:
alpha = random.uniform(0.0, 1.0)
child_1[0] = alpha * parent_1[0] + (1 - alpha) * parent_2[0]
child_1[1] = alpha * parent_1[1] + (1 - alpha) * parent_2[1]
child_2[0] = alpha * parent_2[0] + (1 - alpha) * parent_1[0]
child_2[1] = alpha * parent_2[1] + (1 - alpha) * parent_1[1]
return child_1, child_2
def mutate(self, individual):
child = copy.deepcopy(individual)
gene_index = random.randint(0, len(individual) - 1)
child[gene_index] = random.uniform(0.0, 1.0)
return child
def fitness_function(individual):
return [individual[0] ** 2, (individual[1] - 0.5) ** 2]
nsga2 = NSGA2(fitness_function, 100, 100, 0.9, 0.1)
population = nsga2.run()
print(population)
```
这段代码实现了一个简单的NSGA2算法。在NSGA2类中,fitness_function代表个体的适应度函数,num_generations代表遗传算法的迭代次数,population_size代表种群大小,crossover_prob代表交叉概率,mutation_prob代表变异概率。run函数是NSGA2算法的主函数,它首先初始化种群,然后迭代一定次数,每次进行快速非支配排序,然后根据支配关系生成子代种群,并进行交叉和变异,最后更新种群。initialize_population函数是种群的初始化函数,它实现了生成随机个体的过程。fast_non_dominated_sort函数是快速非支配排序的实现,用于将种群分成一系列前沿等级。is_dominant函数用于判断一个个体是否支配另一个个体。tournament_selection函数是锦标赛选择的实现,用于选择一个个体作为父代。crossover函数是交叉函数的实现,用于生成两个子代。mutate函数是变异函数的实现,用于对个体进行变异。fitness_function是简单的适应度函数,它返回个体两个维度的平方和作为适应度值。
以上就是一段实现NSGA2算法的Python代码。
相关推荐
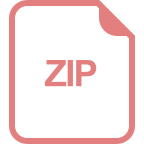
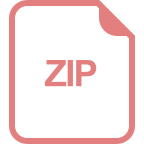
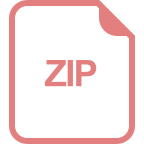













