代码实现最佳缝合线算法
时间: 2023-07-26 16:05:30 浏览: 153
最佳缝合线算法(Seam Carving)是一种图像处理算法,用于在保持图像宽高比的情况下,缩小或扩大图像的大小。
以下是实现最佳缝合线算法的代码:
1. 计算能量值
```python
import numpy as np
import cv2
def energy(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
sobel_x = cv2.Sobel(gray, cv2.CV_64F, 1, 0, ksize=3)
sobel_y = cv2.Sobel(gray, cv2.CV_64F, 0, 1, ksize=3)
return np.abs(sobel_x) + np.abs(sobel_y)
```
2. 计算最小能量路径
```python
def find_seam(image):
e = energy(image)
m = np.zeros_like(e)
m[0] = e[0]
for i in range(1, m.shape[0]):
for j in range(m.shape[1]):
if j == 0:
m[i][j] = e[i][j] + min(m[i-1][j], m[i-1][j+1])
elif j == m.shape[1]-1:
m[i][j] = e[i][j] + min(m[i-1][j], m[i-1][j-1])
else:
m[i][j] = e[i][j] + min(m[i-1][j-1], m[i-1][j], m[i-1][j+1])
seam = []
j = np.argmin(m[-1])
seam.append((len(e)-1, j))
for i in reversed(range(len(e)-1)):
if j == 0:
j = np.argmin(m[i][j:j+2])
elif j == m.shape[1]-1:
j = np.argmin(m[i][j-1:j+1]) + j - 1
else:
j = np.argmin(m[i][j-1:j+2]) + j - 1
seam.append((i, j))
return seam[::-1]
```
3. 删除最小能量路径
```python
def remove_seam(image, seam):
h, w = image.shape[:2]
mask = np.ones((h, w), dtype=np.bool)
for i, j in seam:
mask[i, j] = False
return image[mask].reshape((h, w-1, 3))
```
4. 缩小图像
```python
def resize(image, size):
h, w = image.shape[:2]
dy, dx = h - size[1], w - size[0]
if dx > 0:
for i in range(dx):
seam = find_seam(image)
image = remove_seam(image, seam)
if dy > 0:
image = np.rot90(image, 1, (0, 1))
for i in range(dy):
seam = find_seam(image)
image = remove_seam(image, seam)
image = np.rot90(image, 3, (0, 1))
return image
```
上述代码实现了最佳缝合线算法,可以通过调用 `resize` 函数对图片进行缩放。
阅读全文
相关推荐
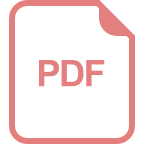
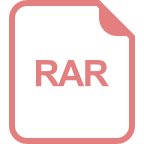
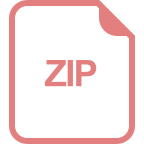










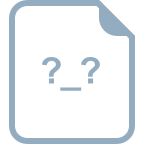
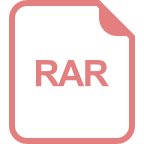
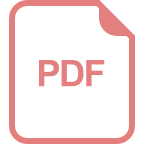
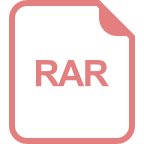
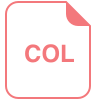
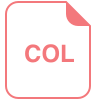