请根据以下代码为模版改写一个Dijkstra单元最短路的堆优化版,其中和堆有关的函数要提供相关代码并注释。 bool Dijkstra(LGraph G, int dist[], int path[], Vertex S) { Vertex V = Error; AdjNode W = G->Graph[S].FirstEdge; while (W) { dist[W->AdjV] = W->Weight; path[W->AdjV] = S; W = W->Next; } dist[S] = 0; collected[S] = true; while (1) { V = FindMinDist(G, dist, collected); if (V == Error) break; else { collected[V] = true; W = G->Graph[V].FirstEdge; while (W) { if (collected[W->AdjV] == false) { if (W->Weight < 0) return false; else { if (dist[V] + W->Weight < dist[W->AdjV]) { dist[W->AdjV] = dist[V] + W->Weight; path[W->AdjV] = V; } } } W = W->Next; } } } return true; } 堆的定义和初始化,注意这是一个定义了卫兵的最小堆 #define MinData -1000 struct HeapStruct { ElementType *Elements; int Size; int Capacity; }; typedef struct HeapStruct *MinHeap; MinHeap CreateHeap(int MaxSize) { MinHeap H = (MinHeap)malloc(sizeof(struct HeapStruct)); H->Elements = (ElementType *)malloc((MaxSize + 1) * sizeof(ElementType)); H->Elements[0] = MinData; H->Size = 0; H->Capacity = MaxSize; return H; }
时间: 2024-02-14 07:29:59 浏览: 19
以下是堆优化版的Dijkstra单元最短路算法的改写:
bool Dijkstra(LGraph G, int dist[], int path[], Vertex S)
{
Vertex V;
AdjNode W;
bool *collected = (bool *)malloc((G->Nv + 1) * sizeof(bool));
MinHeap H = CreateHeap(G->Nv);
// 初始化距离和路径数组
for (V = 0; V < G->Nv; V++)
{
dist[V] = INT_MAX;
path[V] = -1;
collected[V] = false;
}
dist[S] = 0;
// 将起点S插入堆中
Insert(H, S, dist);
while (!IsEmpty(H))
{
// 取出堆中最小距离顶点
V = DeleteMin(H, dist);
collected[V] = true;
// 遍历V的邻接点W
W = G->Graph[V].FirstEdge;
while (W)
{
if (!collected[W->AdjV])
{
if (W->Weight < 0)
return false;
else
{
// 如果经过V到达W的路径更短,则更新距离和路径数组
if (dist[V] + W->Weight < dist[W->AdjV])
{
dist[W->AdjV] = dist[V] + W->Weight;
path[W->AdjV] = V;
// 更新堆中W的距离值
DecreaseKey(H, W->AdjV, dist);
}
}
}
W = W->Next;
}
}
free(collected);
DestroyHeap(H);
return true;
}
其中,Insert函数用于将顶点V和对应的距离dist插入堆中,DeleteMin函数用于删除堆中最小距离顶点,并返回该顶点的值,DecreaseKey函数用于更新堆中某个顶点的距离值。这些函数的实现可以根据堆的定义和初始化代码进行编写,这里不再重复给出。
相关推荐
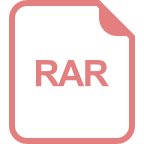














