如何用C++代码计算高精度整数幂?
时间: 2024-10-05 12:01:16 浏览: 11
在C++中,计算高精度整数幂可以使用递归法或者牛顿迭代法。这里我们以递归法为例来展示如何实现:
```cpp
#include <iostream>
#include <string>
// 定义大整数数据结构
template<typename IntegerType>
class BigInt {
public:
BigInt() : value(0) {}
BigInt(const std::string &str) { fromString(str); }
BigInt(IntegerType val) : value(val) {}
private:
IntegerType value;
// 辅助函数将字符串转换为大整数
void fromString(const std::string &str) {
value = 0;
for (const char c : str) {
value *= 10;
value += c - '0';
}
}
// 辅助函数用于高精度乘法
BigInt multiply(const BigInt &other) const {
BigInt result = BigInt(value);
return result *= other;
}
// 高精度乘方递归函数
BigInt pow(const BigInt &base, const BigInt &exponent) const {
if (exponent.value == 0) return BigInt(1);
if (exponent.value % 2 == 0) {
BigInt half = pow(base, exponent.value / 2);
return half.multiply(half);
} else {
return base.pow(exponent.value - 1).multiply(base);
}
}
};
int main() {
BigInt<std::int64_t> a("12345678901234567890"), b("2");
BigInt<std::int64_t> result = a.pow(b);
std::cout << "Result: " << result.value << std::endl;
return 0;
}
```
这个例子中,`BigInt`是一个模板类,它支持基本的加减乘除操作以及高精度的乘方运算。在`pow`函数中,通过递归处理了指数为偶数和奇数两种情况。
相关推荐
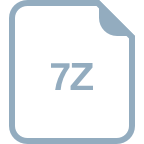
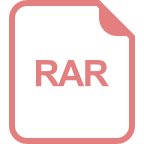
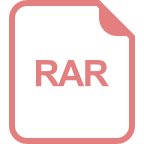
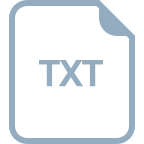
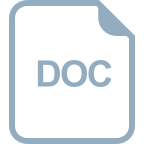

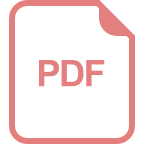
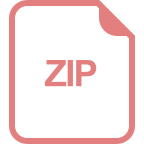
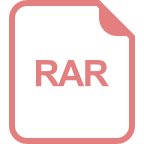
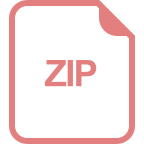
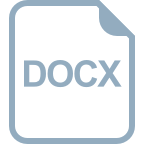
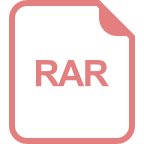
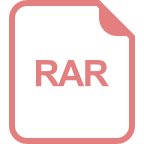
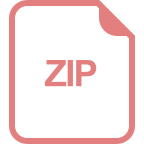
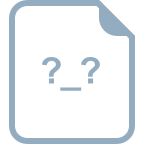
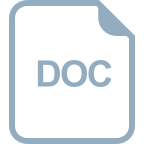
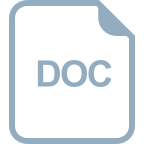
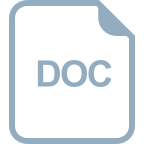
