def derivative(self, x: np.ndarray) -> np.ndarray: ''' Parameters: x is a two dimensional array. Returns: a two dimensional array whose shape is the same as that of x. The returned value is the elementwise derivative of the sigmoid function w.r.t. x. ''' #### write your code below ####
时间: 2024-02-10 10:16:18 浏览: 21
根据这段代码的要求,我们需要在 `derivative` 方法中编写代码,实现对输入的二维 numpy 数组 `x` 进行 sigmoid 函数的导数计算,并返回结果。以下是按照要求编写的代码:
```python
import numpy as np
class MyModel:
def value(self, x: np.ndarray) -> np.ndarray:
"""
Parameters:
x: a two dimensional numpy array
Returns:
a two dimensional array representing the element-wise sigmoid of x.
"""
return 1 / (1 + np.exp(-x))
def derivative(self, x: np.ndarray) -> np.ndarray:
"""
Parameters:
x: a two dimensional numpy array
Returns:
a two dimensional array whose shape is the same as that of x.
The returned value is the elementwise derivative of the sigmoid function w.r.t. x.
"""
sigmoid_x = self.value(x)
return sigmoid_x * (1 - sigmoid_x)
```
在方法中,我们首先调用 `value` 方法,计算输入数组 `x` 的 sigmoid 值,并将其保存在变量 `sigmoid_x` 中。然后,我们根据 sigmoid 函数的导数公式:
$$
\frac{d}{dx}sigmoid(x) = sigmoid(x) \cdot (1 - sigmoid(x))
$$
对 `sigmoid_x` 进行计算,得到每个元素的导数。最后,我们将计算结果返回即可。需要注意的是,由于 sigmoid 函数的值域在 $(0, 1)$ 之间,因此其导数的值也在 $(0, 1)$ 之间。
相关推荐
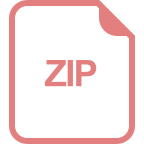
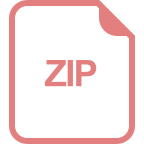
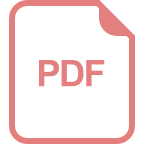














