设一个线性表采用头结点的单链表保存,设计一个算法重新排列该线性表中的各节点完整代码
时间: 2024-10-19 16:05:12 浏览: 27
为了重新排列线性表(单链表)中的节点,我们通常可以使用归并排序的思想,将链表拆分为两半,然后递归地对这两半进行排序,最后合并它们。以下是使用Python实现的一个简单示例:
```python
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge_sort(head):
# 如果链表长度小于等于1,已经有序,直接返回
if head is None or head.next is None:
return head
# 找到中间节点
slow, fast = head, head.next
while fast and fast.next:
slow = slow.next
fast = fast.next.next
# 分割链表
mid = slow.next
slow.next = None
# 对左右两个部分分别递归排序
left = merge_sort(head)
right = merge_sort(mid)
# 合并两个已排序的部分
return merge(left, right)
def merge(left, right):
dummy = ListNode(0) # 创建虚拟头节点
current = dummy
while left and right:
if left.val < right.val:
current.next = left
left = left.next
else:
current.next = right
right = right.next
current = current.next
# 链表未遍历完的部分添加到最后
if left:
current.next = left
elif right:
current.next = right
return dummy.next
# 示例使用
# 假设我们有一个链表,例如 [4, 2, 1, 5, 3]
head = ListNode(4)
head.next = ListNode(2)
head.next.next = ListNode(1)
head.next.next.next = ListNode(5)
head.next.next.next.next = ListNode(3)
sorted_head = merge_sort(head)
```
这个代码首先实现了`ListNode`类表示链表节点,接着定义了归并排序的`merge_sort`函数和合并两个已排序链表的`merge`函数。最后创建了一个链表实例,并应用`merge_sort`对其进行重新排列。
如果你需要了解其他语言(如C++或JavaScript)的版本,我可以帮你提供相应的代码。
阅读全文
相关推荐
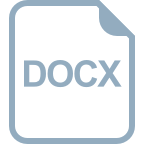
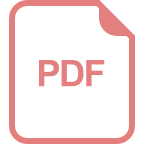
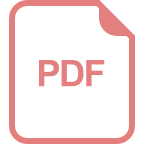
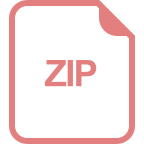
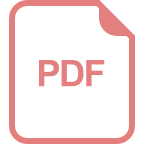
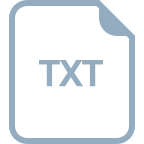
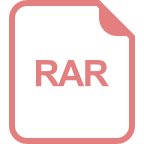
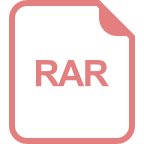
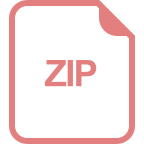
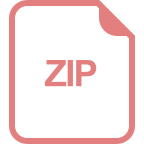
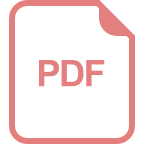
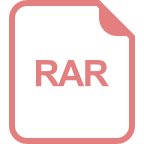
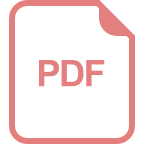
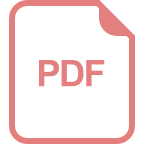
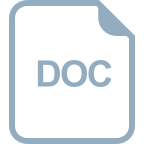
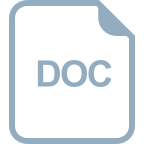
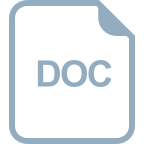