c语言获取当前线程id
时间: 2024-10-25 20:09:18 浏览: 4
在C语言中,直接获取当前线程ID并不像其他高级语言那样直接可用,因为C语言本身并不支持原生的线程管理。不过,如果你正在使用的是支持POSIX标准的操作系统(如Linux),你可以通过`pthread`库来间接实现这个功能。
首先,你需要包含头文件`pthread.h`:
```c
#include <pthread.h>
```
然后,可以使用`pthread_self()`函数来获取当前线程的ID,该函数返回一个`pthread_t`类型的值,这是线程标识符,通常表示为一个无符号长整型。这里是一个简单的示例:
```c
pthread_t thread_id;
// 创建并启动一个新的线程
if (pthread_create(&thread_id, NULL, my_thread_function, NULL) != 0) {
// 处理错误
}
// 现在你可以打印出线程ID
printf("Current thread ID: %lu\n", (unsigned long)thread_id);
```
请记住,为了能访问到线程ID,需要在创建线程之前初始化它。
相关问题
C语言三个线程循环打印ABC
在C语言中,可以使用`pthread`库来实现多线程。具体代码如下:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t a_lock, b_lock, c_lock;
void* print_thread(void* arg) {
int id = *(int*)arg;
int i;
for (i = 0; i < 5; i++) {
if (id == 0) {
pthread_mutex_lock(&c_lock);
printf("A");
pthread_mutex_unlock(&a_lock);
} else if (id == 1) {
pthread_mutex_lock(&a_lock);
printf("B");
pthread_mutex_unlock(&b_lock);
} else {
pthread_mutex_lock(&b_lock);
printf("C");
pthread_mutex_unlock(&c_lock);
}
}
pthread_exit(NULL);
}
int main() {
pthread_t threads[3];
int ids[3] = {0, 1, 2};
pthread_mutex_init(&a_lock, NULL);
pthread_mutex_init(&b_lock, NULL);
pthread_mutex_init(&c_lock, NULL);
pthread_mutex_lock(&a_lock);
pthread_mutex_lock(&b_lock);
pthread_create(&threads[0], NULL, print_thread, &ids[0]);
pthread_create(&threads[1], NULL, print_thread, &ids[1]);
pthread_create(&threads[2], NULL, print_thread, &ids[2]);
pthread_join(threads[0], NULL);
pthread_join(threads[1], NULL);
pthread_join(threads[2], NULL);
pthread_mutex_destroy(&a_lock);
pthread_mutex_destroy(&b_lock);
pthread_mutex_destroy(&c_lock);
return 0;
}
```
其中,定义了一个`print_thread`函数,用于执行打印操作。每个线程需要持有前一个线程的锁和自己的锁,当前一个线程执行完后,释放前一个锁,再获取自己的锁,进行打印操作。最后,主线程等待所有线程执行完毕后,销毁锁并退出程序。
c语言 创建一个线程,该线程输出自己的线程ID
在 C 语言中,创建线程通常使用POSIX标准的`pthread`库。下面是一个简单的示例,展示了如何创建一个新线程并让它打印出当前线程的 ID:
```c
#include <stdio.h>
#include <pthread.h>
// 线程函数,将作为参数传递给 pthread_create()
void *thread_func(void *arg) {
pthread_t thread_id; // 存储当前线程ID的变量
pthread_getspecific(pthread_self); // 获取当前线程ID
printf("Current Thread ID: %lu\n", (unsigned long)pthread_self()); // 输出线程ID
return NULL;
}
int main() {
pthread_t my_thread; // 新建一个线程标识符
int rc;
// 创建一个新的线程并传入 thread_func 函数作为目标
rc = pthread_create(&my_thread, NULL, &thread_func, NULL);
if (rc != 0) {
perror("Error creating thread");
exit(1);
}
// 主线程执行其他操作...
// 这里为了简化例子,我们暂时不做其他事情
// 等待新创建的线程结束
pthread_join(my_thread, NULL);
return 0;
}
```
在这个示例中,`thread_func`是线程要执行的函数,它会获取并打印当前线程ID。`pthread_create`函数用于创建新线程,`pthread_join`用于等待线程完成。
阅读全文
相关推荐
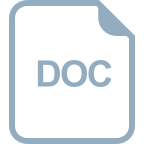
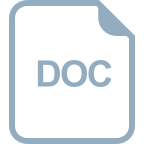
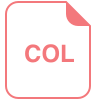
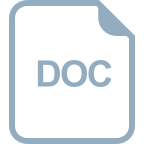
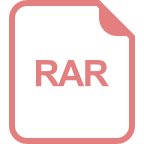
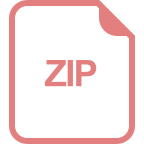
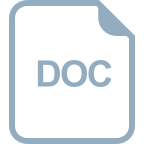
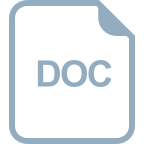
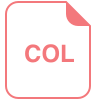
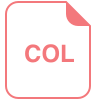
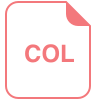
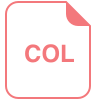




