双线性插值代码python
时间: 2023-11-12 22:58:11 浏览: 98
好的,以下是双线性插值的 Python 代码:
```python
import numpy as np
def bilinear_interpolation(img, x, y):
x1 = int(x)
x2 = x1 + 1
y1 = int(y)
y2 = y1 + 1
if x2 >= img.shape[1]:
x2 = x1
if y2 >= img.shape[0]:
y2 = y1
Q11 = img[y1, x1]
Q21 = img[y1, x2]
Q12 = img[y2, x1]
Q22 = img[y2, x2]
fxy1 = (x2 - x) / (x2 - x1) * Q11 + (x - x1) / (x2 - x1) * Q21
fxy2 = (x2 - x) / (x2 - x1) * Q12 + (x - x1) / (x2 - x1) * Q22
fxy = (y2 - y) / (y2 - y1) * fxy1 + (y - y1) / (y2 - y1) * fxy2
return fxy
```
其中,`img` 是输入的图像,`x` 和 `y` 是需要插值的位置坐标。函数返回在该位置的插值结果。
相关问题
双线性插值公式Python
双线性插值是一种常见的数值插值方法,在计算机图形学、图像处理等领域广泛应用。当需要对二维数据集进行估算时,如果给定的是四个已知点的坐标值和它们对应的值,可以使用双线性插值公式来获取中间点的值。
假设我们有四个点 (x0, y0)、(x1, y1), (x2, y2), 和 (x3, y3),并且需要计算位于 x0 和 x1 之间的 y 值,以及 y0 和 y1 之间的 x 值。双线性插值公式如下:
对于 x 的插值(假设 x 在 [x0, x1] 范围内):
```python
y_interpolated = ((x - x0) + y1
```
对于 y 的插值(同样假设 y 在 [y0, y1] 范围内):
```python
x_interpolated = ((y - y0) * (x2 - x0)) / (y1 - y0) + x0
```
在Python中,你可以创建一个简单的函数来实现这个过程:
```python
def bilinear_interpolation(x, y, points):
x0, y0, x1, y1 = points
if x <= x0 and y <= y0:
return x0, y0
elif x > x1 or y > y1:
return x1, y1
else:
u = (x - x0) / (x1 - x0)
v = (y - y0) / (y1 - y0)
x_interpolated = x0 + u * (x1 - x0)
y_interpolated = y0 + v * (y1 - y0)
return x_interpolated, y_interpolated
# 使用示例
points = [(0, 0), (1, 1), (2, 2), (3, 3)]
x = 1.5
y = 1.5
interpolated_point = bilinear_interpolation(x, y, points)
print(f"Interpolated point at ({x}, {y}): ({interpolated_point[0]}, {interpolated_point[1]})")
```
双线性插值算法python
双线性插值算法的Python实现如下:
```python
from PIL import Image
import numpy as np
import math
def BiLinear_interpolation(img, dstH, dstW):
scrH, scrW, _ = img.shape
img = np.pad(img, ((0, 1), (0, 1), (0, 0)), 'constant')
retimg = np.zeros((dstH, dstW, 3), dtype=np.uint8)
for i in range(dstH):
for j in range(dstW):
scrx = (i + 1) * (scrH / dstH) - 1
scry = (j + 1) * (scrW / dstW) - 1
x = math.floor(scrx)
y = math.floor(scry)
u = scrx - x
v = scry - y
retimg\[i, j\] = (1 - u) * (1 - v) * img\[x, y\] + u * (1 - v) * img\[x + 1, y\] + (1 - u) * v * img\[x, y + 1\] + u * v * img\[x + 1, y + 1\]
return retimg
im_path = 'wave.jpg'
image = np.array(Image.open(im_path))
image2 = BiLinear_interpolation(image, image.shape\[0\] * 2, image.shape\[1\] * 2)
image2 = Image.fromarray(image2.astype('uint8')).convert('RGB')
image2.save('out.jpg')
```
这段代码使用了PIL库和numpy库,首先读取了一张图片,然后调用了`BiLinear_interpolation`函数进行双线性插值算法的处理。该函数接受原始图像、目标图像的高度和宽度作为参数,返回处理后的图像。最后,将处理后的图像保存为`out.jpg`。
#### 引用[.reference_title]
- *1* *2* [Python双线性插值(Bilinear Interpolation)(一) —— 原理 & 手动实现 (最近邻插值 & 双三次插值)](https://blog.csdn.net/hxxjxw/article/details/118229375)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [双线性插值(python实现)](https://blog.csdn.net/Spratumn/article/details/103583237)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
阅读全文
相关推荐
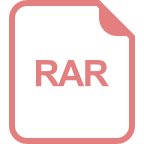












