写一个图像双线性插值python代码
时间: 2024-02-01 10:04:46 浏览: 30
好的,以下是双线性插值的 Python 代码示例:
```python
import numpy as np
from PIL import Image
def bilinear_interpolation(img, x, y):
"""双线性插值"""
x1 = int(x)
y1 = int(y)
x2 = x1 + 1
y2 = y1 + 1
# 计算四个点的灰度值
f11 = img[y1][x1]
f12 = img[y1][x2]
f21 = img[y2][x1]
f22 = img[y2][x2]
# 插值计算
result = (
f11 * (x2 - x) * (y2 - y) +
f12 * (x - x1) * (y2 - y) +
f21 * (x2 - x) * (y - y1) +
f22 * (x - x1) * (y - y1)
)
return result
def resize_bilinear(img, height, width):
"""双线性插值图像缩放"""
old_height, old_width = img.shape
# x轴和y轴上采样率
x_ratio = old_width / width
y_ratio = old_height / height
# 新建新的数组
new_img = np.zeros((height, width), dtype=np.uint8)
for y in range(height):
for x in range(width):
x_old = x * x_ratio
y_old = y * y_ratio
new_img[y][x] = bilinear_interpolation(img, x_old, y_old)
return new_img
# 加载图像
img = np.array(Image.open('example.jpg').convert('L'))
# 缩放图像
new_img = resize_bilinear(img, 512, 512)
# 保存图像
Image.fromarray(new_img).save('example_resized.jpg')
```
以上代码实现了双线性插值缩放图像的功能。您可以使用Pillow库来加载和保存图像。
相关推荐
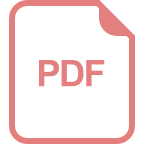
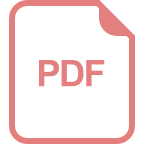
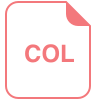
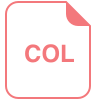
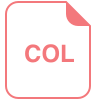











