写一个图像双线性插值代码
时间: 2024-05-15 19:17:24 浏览: 15
以下是一个Python实现的双线性插值代码,你可以输入你需要插值的图像和目标大小,然后程序就会返回插值后的图像。
```python
import numpy as np
from scipy.interpolate import interp2d
def bilinear_interpolation(img, new_size):
height, width = img.shape
new_height, new_width = new_size
x_ratio = float(width - 1) / (new_width - 1)
y_ratio = float(height - 1) / (new_height - 1)
x, y = np.linspace(0, width - 1, num=width), np.linspace(0, height - 1, num=height)
f = interp2d(x, y, img)
new_x = np.arange(new_width)
new_y = np.arange(new_height)
new_x, new_y = np.meshgrid(new_x, new_y)
new_x, new_y = new_x * x_ratio, new_y * y_ratio
return f(new_x, new_y)
# 使用示例,假设现有一个128x128的图像,需要插值成256x256的图像
img = np.random.rand(128, 128)
new_size = (256, 256)
result = bilinear_interpolation(img, new_size)
```
需要注意的是,这个代码只是一个双线性插值的实现,并不是最优化的实现方式。如果需要更快的插值速度或更高的插值质量,建议使用现成库或算法实现。
相关推荐
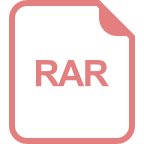
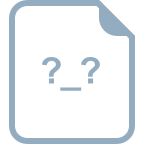
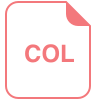
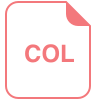
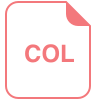











