Write the following code in c #1. Write a method with the following specifications: name: DisplayMenu arguments: none return value: none tasks: display the following menu choice on the screen Calculation Menu, developed by StudentID:xxxx B) Calculate Number E) To Exit Enter the Letter that corresponds to your choice: Please update your ID in the menu. You don’t have to implement the functionalities of the various menu choices at this stage Call this method from your main. 2. Modify your main so that the above method is call repeatedly. The program will terminate when the user enters E. Any other choice should produce an error message. Because you will not be doing any arithmetic you may accept the user response either as an int, or a char or a string. 3. Write a method with the following specifications: name: CalculateN arguments: an int array with values ranging from 0 to 10 return value: int array of 3 integers. The first element will indicate the total sum of all the values in the array, the second element will indicate the average of all the values in the array, the third element will indicate the smallest value in the array. displays: none tasks: The method will create an int array of 3 elements (call this the result). In your main you will generate an int array of 5 elements with values ranging from 0 to 10, call this method and display the resulting array’s value
时间: 2024-01-31 10:02:06 浏览: 64
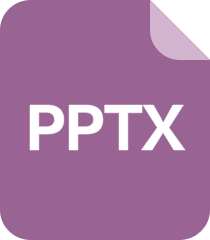
C程序设计教学课件:CHAPTER4FUNCTIONS.pptx
Sure, here's the code in C#:
```csharp
using System;
class Program
{
static void Main(string[] args)
{
char choice;
int[] arr = new int[] { 5, 2, 9, 7, 3 };
do
{
DisplayMenu();
choice = Console.ReadKey().KeyChar;
Console.WriteLine();
switch (choice)
{
case 'B':
int[] result = CalculateN(arr);
Console.WriteLine($"Total sum: {result[0]}");
Console.WriteLine($"Average: {result[1]}");
Console.WriteLine($"Smallest value: {result[2]}");
break;
case 'E':
Console.WriteLine("Exiting...");
break;
default:
Console.WriteLine("Invalid choice. Please try again.");
break;
}
Console.WriteLine();
} while (choice != 'E');
}
static void DisplayMenu()
{
Console.WriteLine("Calculation Menu, developed by StudentID:xxxx");
Console.WriteLine("B) Calculate Number");
Console.WriteLine("E) To Exit");
Console.Write("Enter the Letter that corresponds to your choice: ");
}
static int[] CalculateN(int[] arr)
{
int totalSum = 0;
int smallestValue = arr[0];
foreach (int num in arr)
{
totalSum += num;
if (num < smallestValue)
{
smallestValue = num;
}
}
int average = totalSum / arr.Length;
return new int[] { totalSum, average, smallestValue };
}
}
```
Explanation:
- We start by declaring a `char` variable called `choice` to store the user's menu choice, and an `int` array called `arr` with 5 elements and some sample values.
- In the `Main` method, we use a `do-while` loop to repeatedly display the menu and prompt the user for a choice, until they enter 'E' to exit.
- Inside the loop, we use a `switch` statement to check the user's choice and perform the appropriate action. If they choose 'B', we call the `CalculateN` method with the `arr` array as argument, and display the resulting array's values. If they choose 'E', we simply print a message and the loop will exit.
- The `DisplayMenu` method simply prints the menu to the console.
- The `CalculateN` method takes an `int` array as argument, and returns another `int` array with 3 elements: the total sum, the average, and the smallest value. We use a `foreach` loop to iterate over the array and calculate these values, and then return them as an array.
阅读全文
相关推荐
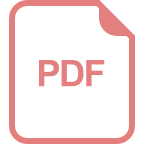
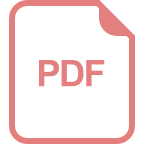















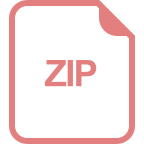