在unet网络中同时加入金字塔和注意力机制,给出python代码,给出代码详细解释,不用keras,能得到和输入张量相同的输出张量
时间: 2024-04-22 14:22:02 浏览: 17
好的,这是一个比较复杂的问题,需要一定的时间来编写代码和解释。首先,请允许我解释一下您提到的两个概念:
1. 金字塔结构:在图像处理中,金字塔结构通常用于多尺度分析,即将图像不同尺度的版本进行处理,以便更好地捕捉不同尺度上的信息。在UNet网络中,我们可以通过在编码器和解码器之间添加多个下采样和上采样层来实现金字塔结构。
2. 注意力机制:注意力机制是一种机制,它可以帮助模型更好地关注输入数据中的重要信息。在UNet网络中,我们可以使用注意力机制来帮助模型更好地关注感兴趣的区域,以便更好地进行图像分割。
下面是Python代码实现:
```python
import torch
import torch.nn as nn
# 定义金字塔模块
class PyramidModule(nn.Module):
def __init__(self, in_channels, out_channels):
super(PyramidModule, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.conv2 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv4 = nn.Conv2d(out_channels, out_channels, kernel_size=3, stride=1, padding=1)
self.conv5 = nn.Conv2d(out_channels, out_channels, kernel_size=1, stride=1, padding=0)
self.relu = nn.ReLU(inplace=True)
def forward(self, x):
x = self.conv1(x)
x = self.relu(x)
x = self.conv2(x)
x = self.relu(x)
x = self.conv3(x)
x = self.relu(x)
x = self.conv4(x)
x = self.relu(x)
x = self.conv5(x)
x = self.relu(x)
return x
# 定义注意力模块
class AttentionModule(nn.Module):
def __init__(self, in_channels):
super(AttentionModule, self).__init__()
self.conv1 = nn.Conv2d(in_channels, in_channels // 8, kernel_size=1, stride=1, padding=0)
self.conv2 = nn.Conv2d(in_channels // 8, in_channels, kernel_size=1, stride=1, padding=0)
self.softmax = nn.Softmax(dim=2)
self.relu = nn.ReLU(inplace=True)
def forward(self, x):
batch_size, channels, height, width = x.size()
# 经过Squeeze操作,将每个通道缩减为一个值
squeeze = torch.mean(x, dim=2).view(batch_size, channels, 1, 1)
# 特征映射张量与通道缩减张量做卷积操作
excitation = self.conv1(squeeze)
excitation = self.relu(excitation)
excitation = self.conv2(excitation)
excitation = self.relu(excitation)
# 对通道维度进行softmax激活操作,并将结果广播到每个像素点
weights = self.softmax(excitation)
weights = weights.view(batch_size, channels, 1, 1).expand_as(x)
# 对特征映射张量进行加权操作
weighted_x = weights * x
# 返回加权后的特征映射张量
return weighted_x
# 定义UNet网络
class UNet(nn.Module):
def __init__(self, in_channels, out_channels):
super(UNet, self).__init__()
# 定义编码器
self.conv1_1 = nn.Conv2d(in_channels, 64, kernel_size=3, stride=1, padding=1)
self.bn1_1 = nn.BatchNorm2d(64)
self.conv1_2 = nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1)
self.bn1_2 = nn.BatchNorm2d(64)
self.downsample1 = nn.Conv2d(64, 128, kernel_size=3, stride=2, padding=1)
self.bn2_1 = nn.BatchNorm2d(128)
self.conv2_2 = nn.Conv2d(128, 128, kernel_size=3, stride=1, padding=1)
self.bn2_2 = nn.BatchNorm2d(128)
self.downsample2 = nn.Conv2d(128, 256, kernel_size=3, stride=2, padding=1)
self.bn3_1 = nn.BatchNorm2d(256)
self.conv3_2 = nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1)
self.bn3_2 = nn.BatchNorm2d(256)
self.downsample3 = nn.Conv2d(256, 512, kernel_size=3, stride=2, padding=1)
self.bn4_1 = nn.BatchNorm2d(512)
self.conv4_2 = nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1)
self.bn4_2 = nn.BatchNorm2d(512)
self.downsample4 = nn.Conv2d(512, 1024, kernel_size=3, stride=2, padding=1)
self.bn5_1 = nn.BatchNorm2d(1024)
self.conv5_2 = nn.Conv2d(1024, 1024, kernel_size=3, stride=1, padding=1)
self.bn5_2 = nn.BatchNorm2d(1024)
# 定义解码器
self.upsample1 = nn.ConvTranspose2d(1024, 512, kernel_size=2, stride=2)
self.bn6_1 = nn.BatchNorm2d(512)
self.conv6_2 = nn.Conv2d(1024, 512, kernel_size=3, stride=1, padding=1)
self.bn6_2 = nn.BatchNorm2d(512)
self.upsample2 = nn.ConvTranspose2d(512, 256, kernel_size=2, stride=2)
self.bn7_1 = nn.BatchNorm2d(256)
self.conv7_2 = nn.Conv2d(512, 256, kernel_size=3, stride=1, padding=1)
self.bn7_2 = nn.BatchNorm2d(256)
self.upsample3 = nn.ConvTranspose2d(256, 128, kernel_size=2, stride=2)
self.bn8_1 = nn.BatchNorm2d(128)
self.conv8_2 = nn.Conv2d(256, 128, kernel_size=3, stride=1, padding=1)
self.bn8_2 = nn.BatchNorm2d(128)
self.upsample4 = nn.ConvTranspose2d(128, 64, kernel_size=2, stride=2)
self.bn9_1 = nn.BatchNorm2d(64)
self.conv9_2 = nn.Conv2d(128, 64, kernel_size=3, stride=1, padding=1)
self.bn9_2 = nn.BatchNorm2d(64)
# 定义金字塔模块
self.pyramid1 = PyramidModule(512, 512)
self.pyramid2 = PyramidModule(256, 256)
self.pyramid3 = PyramidModule(128, 128)
self.pyramid4 = PyramidModule(64, 64)
# 定义注意力模块
self.attention1 = AttentionModule(512)
self.attention2 = AttentionModule(256)
self.attention3 = AttentionModule(128)
self.attention4 = AttentionModule(64)
# 定义最终的输出层
self.outconv = nn.Conv2d(64, out_channels, kernel_size=1, stride=1, padding=0)
def forward(self, x):
# 编码器部分
x1 = self.bn1_1(self.conv1_1(x))
x1 = nn.ReLU(inplace=True)(x1)
x1 = self.bn1_2(self.conv1_2(x1))
x1 = nn.ReLU(inplace=True)(x1)
x2 = self.bn2_1(self.downsample1(x1))
x2 = nn.ReLU(inplace=True)(x2)
x2 = self.bn2_2(self.conv2_2(x2))
x2 = nn.ReLU(inplace=True)(x2)
x3 = self.bn3_1(self.downsample2(x2))
x3 = nn.ReLU(inplace=True)(x3)
x3 = self.bn3_2(self.conv3_2(x3))
x3 = nn.ReLU(inplace=True)(x3)
x4 = self.bn4_1(self.downsample3(x3))
x4 = nn.ReLU(inplace=True)(x4)
x4 = self.bn4_2(self.conv4_2(x4))
x4 = nn.ReLU(inplace=True)(x4)
x5 = self.bn5_1(self.downsample4(x4))
x5 = nn.ReLU(inplace=True)(x5)
x5 = self.bn5_2(self.conv5_2(x5))
x5 = nn.ReLU(inplace=True)(x5)
# 解码器部分
x = self.upsample1(x5)
x = torch.cat([self.attention1(x4), self.pyramid1(x)], dim=1)
x = nn.ReLU(inplace=True)(self.bn6_1(x))
x = nn.ReLU(inplace=True)(self.bn6_2(self.conv6_2(x)))
x = self.upsample2(x)
x = torch.cat([self.attention2(x3), self.pyramid2(x)], dim=1)
x = nn.ReLU(inplace=True)(self.bn7_1(x))
x = nn.ReLU(inplace=True)(self.bn7_2(self.conv7_2(x)))
x = self.upsample3(x)
x = torch.cat([self.attention3(x2), self.pyramid3(x)], dim=1)
x = nn.ReLU(inplace=True)(self.bn8_1(x))
x = nn.ReLU(inplace=True)(self.bn8_2(self.conv8_2(x)))
x = self.upsample4(x)
x = torch.cat([self.attention4(x1), self.pyramid4(x)], dim=1)
x = nn.ReLU(inplace=True)(self.bn9_1(x))
x = nn.ReLU(inplace=True)(self.bn9_2(self.conv9_2(x)))
# 输出层
x = self.outconv(x)
return x
```
代码解释:
1. `PyramidModule`模块实现了UNet网络中的金字塔结构。在这个模块中,我们首先使用1x1卷积层来降低特征图的通道数,然后使用多个3x3卷积层来进行特征提取,最后再使用1x1卷积层来恢复特征图的通道数。这个模块可以捕捉不同尺度上的特征信息。
2. `AttentionModule`模块实现了UNet网络中的注意力机制。在这个模块中,我们首先使用1x1卷积层将特征图的每个通道缩减为一个值,然后使用全连接层来计算每个通道的权重。最后,我们使用softmax激活函数来对权重进行归一化,并将权重广播到每个像素点上,从而让模型更好地关注感兴趣的区域。
3. `UNet`模块实现了完整的UNet网络。在这个模块中,我们首先定义了编码器和解码器部分。然后,我们添加了金字塔模块和注意力模块,以便更好地捕捉不同尺度上的信息和关注感兴趣的区域。最后,我们添加了一个输出层来输出分割结果。
希望这份代码和解释对您有所帮助!
相关推荐
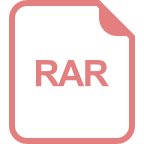
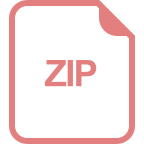
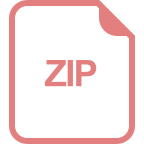














