C++使用Websocket接收和发送json数据
时间: 2024-05-06 08:15:28 浏览: 11
要使用WebSocket在C++中发送和接收JSON数据,您需要使用一个WebSocket客户端库,例如Boost.Beast或uWebSockets。在这里,我将为您提供一个使用Boost.Beast的示例代码。
首先,您需要安装Boost库,并在代码中包含以下头文件:
```cpp
#include <boost/beast/core.hpp>
#include <boost/beast/websocket.hpp>
#include <boost/asio/strand.hpp>
#include <boost/property_tree/json_parser.hpp>
```
接下来,您需要定义一个WebSocket客户端对象和一个Boost.Asio io_context对象:
```cpp
namespace beast = boost::beast;
namespace http = beast::http;
namespace websocket = beast::websocket;
using tcp = boost::asio::ip::tcp;
boost::asio::io_context io_context;
websocket::stream<tcp::socket> websocket_stream(io_context);
```
然后,您需要连接到WebSocket服务器:
```cpp
tcp::resolver resolver(io_context);
auto const results = resolver.resolve("example.com", "80");
boost::asio::connect(websocket_stream.next_layer(), results.begin(), results.end());
websocket_stream.handshake("example.com", "/");
```
现在,您已经连接到WebSocket服务器。接下来,您可以使用以下代码发送JSON数据:
```cpp
boost::property_tree::ptree root;
root.put("key1", "value1");
root.put("key2", "value2");
std::ostringstream oss;
boost::property_tree::write_json(oss, root, false);
websocket_stream.write(boost::asio::buffer(oss.str()));
```
要接收JSON数据,请使用以下代码:
```cpp
beast::multi_buffer buffer;
websocket_stream.read(buffer);
std::string json_str = boost::beast::buffers_to_string(buffer.data());
std::istringstream iss(json_str);
boost::property_tree::ptree root;
boost::property_tree::read_json(iss, root);
std::string value1 = root.get<std::string>("key1");
std::string value2 = root.get<std::string>("key2");
```
这样,您就可以使用WebSocket在C++中发送和接收JSON数据了。请注意,此示例代码仅供参考,您需要根据您的具体情况进行修改。
相关推荐
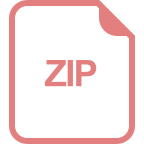
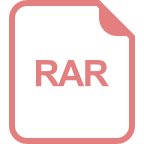
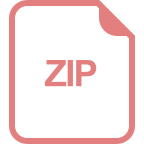









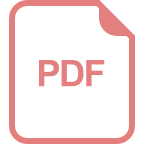
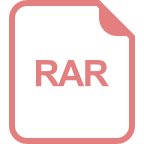
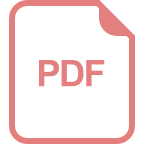
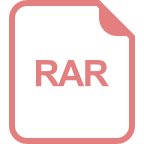