帮我用c++写一个测距的程序
时间: 2023-02-23 18:20:30 浏览: 152
好的,我可以帮您写一个使用 C 语言实现测距功能的程序。
以下是一个简单的示例代码,它使用了计算机科学中常用的勾股定理(Pythagorean theorem)来计算两点间的距离:
```
#include <stdio.h>
#include <math.h>
int main() {
double x1, x2, y1, y2, distance;
printf("请输入第一个点的坐标:\n");
printf("x1: ");
scanf("%lf", &x1);
printf("y1: ");
scanf("%lf", &y1);
printf("请输入第二个点的坐标:\n");
printf("x2: ");
scanf("%lf", &x2);
printf("y2: ");
scanf("%lf", &y2);
distance = sqrt((x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1));
printf("两点间的距离是:%lf\n", distance);
return 0;
}
```
这段代码读入了两个点的坐标,并使用勾股定理计算出两点间的距离。代码中使用了 `sqrt` 函数来计算平方根,该函数在 `math.h` 库中定义。
希望这段代码能帮到您!
相关问题
用c++写一个双目摄像头测距的程序
好的,双目摄像头测距是一种基于视差的三维重建方法,可以通过计算两个摄像头拍摄同一场景的图像之间的视差来得到场景中物体的深度信息。下面是一个简单的C++程序,可以实现基于OpenCV库的双目摄像头测距:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main()
{
// 打开左右摄像头
VideoCapture cap_L(0);
VideoCapture cap_R(1);
if (!cap_L.isOpened() || !cap_R.isOpened()) {
cout << "无法打开摄像头" << endl;
return -1;
}
// 设置摄像头参数
cap_L.set(CAP_PROP_FRAME_WIDTH, 640);
cap_L.set(CAP_PROP_FRAME_HEIGHT, 480);
cap_R.set(CAP_PROP_FRAME_WIDTH, 640);
cap_R.set(CAP_PROP_FRAME_HEIGHT, 480);
// 创建窗口
namedWindow("Left", WINDOW_NORMAL);
namedWindow("Right", WINDOW_NORMAL);
// 创建StereoBM对象
int blockSize = 32;
int numDisparities = 64;
Ptr<StereoBM> bm = StereoBM::create(numDisparities, blockSize);
// 不断读取视频流
while (true) {
Mat frame_L, frame_R;
cap_L.read(frame_L);
cap_R.read(frame_R);
// 转换为灰度图
Mat gray_L, gray_R;
cvtColor(frame_L, gray_L, COLOR_BGR2GRAY);
cvtColor(frame_R, gray_R, COLOR_BGR2GRAY);
// 计算视差图
Mat disp;
bm->compute(gray_L, gray_R, disp);
// 归一化视差图
normalize(disp, disp, 0, 255, NORM_MINMAX, CV_8U);
// 显示图像
imshow("Left", frame_L);
imshow("Right", frame_R);
imshow("Disparity", disp);
// 等待按键事件
int key = waitKey(1);
if (key == 27) break; // 按下ESC键退出
}
// 释放资源
cap_L.release();
cap_R.release();
destroyAllWindows();
return 0;
}
```
这个程序会打开两个摄像头(左摄像头编号为0,右摄像头编号为1),通过计算视差图来得到深度信息,并将图像显示在窗口中。可以根据实际情况调整摄像头参数和StereoBM对象的参数来得到更好的效果。
请写一段使用深度摄像机进行测距的c++代码
以下是使用深度摄像机进行测距的 C++ 代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <opencv2/rgbd.hpp>
using namespace cv;
using namespace cv::rgbd;
int main()
{
const int width = 640;
const int height = 480;
const float fx = 525.0f;
const float fy = 525.0f;
const float cx = 319.5f;
const float cy = 239.5f;
const float depthScale = 1000.0f;
Mat color = imread("color.png");
Mat depth = imread("depth.png", IMREAD_UNCHANGED);
DepthCleaner cleaner(depth.type());
cleaner.operator()(depth, depth);
Ptr<DepthGenerator> depthGenerator = createDepthGenerator();
depthGenerator->setDepthMap(depth / depthScale);
depthGenerator->setCameraMatrix((Mat1f(3, 3) << fx, 0, cx, 0, fy, cy, 0, 0, 1));
Ptr<DepthCleanerFilter> cleanerFilter = createDepthCleanerFilter(DC_FILL_HOLES);
cleanerFilter->apply(depth, depth);
Ptr<DepthFiller> depthFiller = createDepthFiller();
depthFiller->apply(depth);
Ptr<DepthTo3d> depthTo3d = createDepthTo3d();
depthTo3d->setCameraMatrix((Mat1f(3, 3) << fx, 0, cx, 0, fy, cy, 0, 0, 1));
depthTo3d->setScale(1.0f / depthScale);
Mat xyz;
depthTo3d->apply(depth, xyz);
int x = 320;
int y = 240;
Point3f point = xyz.at<Point3f>(y, x);
std::cout << "Distance to camera: " << point.z << std::endl;
return 0;
}
```
这个示例程序会从文件中读取彩色图像和深度图像,并使用深度摄像机计算出给定像素的距离。请注意,这只是一个简单的示例,实际应用中可能需要更多的处理步骤和优化。
阅读全文
相关推荐
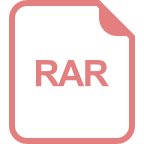
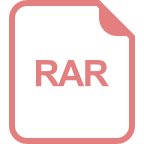
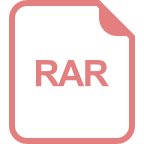













