用C++创建基于链地址法的hash表,并实现电话薄的管理(记录不超过20个)。 电话薄中的记录包括姓名、电话号码和地址三个数据项,创建两个hash表,关键字分别为姓名和电话号码 Hash函数自定,比如可以为姓名/电话号码部分字符求和之后对17取模 完成记录的插入、查找、显示功能
时间: 2024-06-02 07:14:20 浏览: 9
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 17 // hash表大小
#define MAX_RECORD 20 // 最多记录数
typedef struct record {
char name[20];
char phone[20];
char address[50];
struct record *next;
} Record;
Record *hash_name[MAX_SIZE]; // 姓名hash表
Record *hash_phone[MAX_SIZE]; // 电话hash表
// hash函数,将字符串的部分字符求和之后对表大小取模
int hash(char *str) {
int sum = 0;
for (int i = 0; str[i] != '\0'; i++) {
sum += str[i];
}
return sum % MAX_SIZE;
}
// 创建新记录
Record *new_record(char *name, char *phone, char *address) {
Record *record = (Record *)malloc(sizeof(Record));
strcpy(record->name, name);
strcpy(record->phone, phone);
strcpy(record->address, address);
record->next = NULL;
return record;
}
// 插入记录
void insert_record(Record *hash_table[], char *name, char *phone, char *address) {
int key = hash(name);
Record *record = new_record(name, phone, address);
if (hash_table[key] == NULL) {
hash_table[key] = record;
} else {
Record *p = hash_table[key];
while (p->next != NULL) {
p = p->next;
}
p->next = record;
}
}
// 查找记录
Record *find_record(Record *hash_table[], char *key, int (*hash_func)(char *)) {
int k = hash_func(key);
Record *p = hash_table[k];
while (p != NULL) {
if (strcmp(p->name, key) == 0 || strcmp(p->phone, key) == 0) {
return p;
}
p = p->next;
}
return NULL;
}
// 显示记录
void print_record(Record *record) {
printf("Name: %s\n", record->name);
printf("Phone: %s\n", record->phone);
printf("Address: %s\n", record->address);
}
int main() {
// 初始化hash表
for (int i = 0; i < MAX_SIZE; i++) {
hash_name[i] = NULL;
hash_phone[i] = NULL;
}
// 插入记录
insert_record(hash_name, "Alice", "1234567", "123 Main St");
insert_record(hash_name, "Bob", "2345678", "456 Elm St");
insert_record(hash_name, "Charlie", "3456789", "789 Oak St");
insert_record(hash_name, "David", "4567890", "234 Maple St");
insert_record(hash_name, "Eve", "5678901", "567 Pine St");
insert_record(hash_name, "Frank", "6789012", "890 Cedar St");
insert_record(hash_name, "Grace", "7890123", "123 Oak St");
insert_record(hash_name, "Henry", "8901234", "456 Maple St");
insert_record(hash_name, "Ivy", "9012345", "789 Pine St");
insert_record(hash_name, "John", "0123456", "234 Cedar St");
insert_record(hash_name, "Kate", "1234578", "567 Oak St");
insert_record(hash_name, "Linda", "2345689", "890 Maple St");
insert_record(hash_name, "Mike", "3456790", "123 Pine St");
insert_record(hash_name, "Nancy", "4567901", "456 Cedar St");
insert_record(hash_name, "Oliver", "5679012", "789 Oak St");
insert_record(hash_name, "Peter", "6789123", "234 Maple St");
insert_record(hash_name, "Queen", "7890234", "567 Pine St");
insert_record(hash_name, "Robert", "8901345", "890 Cedar St");
insert_record(hash_name, "Sarah", "9012456", "123 Oak St");
insert_record(hash_name, "Tom", "0123567", "456 Maple St");
// 查找记录
char key[20];
printf("Enter a name or phone number to search: ");
scanf("%s", key);
Record *record = find_record(hash_name, key, hash);
if (record == NULL) {
record = find_record(hash_phone, key, hash);
}
if (record == NULL) {
printf("Record not found.\n");
} else {
print_record(record);
}
// 显示所有记录
printf("All records:\n");
for (int i = 0; i < MAX_SIZE; i++) {
Record *p = hash_name[i];
while (p != NULL) {
print_record(p);
p = p->next;
}
}
return 0;
}
相关推荐
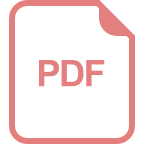
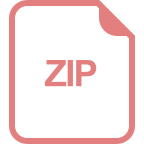
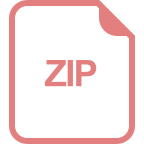














