Python设计程序 输入月份x,判断2023年每个月份的天数
时间: 2023-05-23 21:06:31 浏览: 101
A:可以使用Python中的条件语句if-elif-else来完成该程序。
```
# 输入月份
x = int(input("请输入月份:"))
# 判断月份对应的天数
if x == 1 or x == 3 or x == 5 or x == 7 or x == 8 or x == 10 or x == 12:
days = 31
elif x == 4 or x == 6 or x == 9 or x == 11:
days = 30
elif x == 2:
days = 28
else:
print("输入月份有误!")
days = 0
# 输出结果
if days != 0:
print("2023年", x, "月有", days, "天。")
```
解释:
首先获取输入的月份值x,然后使用if-elif-else语句根据不同月份判断所对应的天数。其中1、3、5、7、8、10、12月有31天,4、6、9、11月有30天,2月有28天;如果输入月份不在1到12之间,则输出提示信息。最后根据判断结果输出2023年对应月份的天数。
相关问题
Python设计程序输入月份x,判断2023年每个月份的天数
A:
```python
def days_in_month(x):
if x in (1, 3, 5, 7, 8, 10, 12):
return 31
elif x in (4, 6, 9, 11):
return 30
elif x == 2:
return 28 if is_leap_year(2023) else 29
def is_leap_year(year):
return (year % 4 == 0 and year % 100 != 0) or year % 400 == 0
for i in range(1, 13):
print("2023年{}月的天数:{}".format(i, days_in_month(i)))
```
或者更简单的方法:
```python
import calendar
for i in range(1, 13):
print("2023年{}月的天数:{}".format(i, calendar.monthrange(2023, i)[1]))
```
使用python代码做一个天数计算小程序 例如用户输入2023年11月1日,程序可以输出这是今年的 第305天 不要引用datetime
你可以通过编写一个简单的Python函数来实现这个功能,这里不使用内置的`datetime`模块,而是手动计算日期之间的差值。我们可以创建两个列表来存储每个月的天数以及闰年的规则。以下是实现这个功能的代码:
```python
def is_leap_year(year):
if year % 4 != 0:
return False
elif year % 100 != 0:
return True
elif year % 400 != 0:
return False
else:
return True
def days_in_month(month, year):
months_days = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if month == 2 and is_leap_year(year):
return 29
else:
return months_days[month - 1]
def calculate_days(date_string):
date_format = "%Y-%m-%d"
input_date = date_string.split("-")
year, month, day = int(input_date[0]), int(input_date[1]), int(input_date[2])
total_days = sum(days_in_month(month, y) for y in range(year, 1))
total_days += day - 1 # 减去开始的0天
return total_days
date_input = input("请输入日期(格式:YYYY-MM-DD),如2023年11月1日:")
days_since_year_start = calculate_days(date_input)
print(f"这是今年的第{days_since_year_start}天")
#
阅读全文
相关推荐
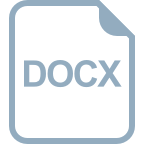
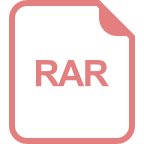
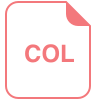










